This recognizes `let a = [1u8, 2, 3]` as having type `[u8; 3]` instead
of the previous `[u8; _]`. Byte strings and `[0u8; 2]` kinds of range
array declarations are unsupported as before.
I don't know why a bunch of our rustc tests had single quotes inside
strings un-escaped by `UPDATE_EXPECT=1 cargo t`, but I don't think it's
bad? Maybe something in a nightly?
8794: Give MergeBehaviour variants better names r=Veykril a=Veykril
I never really liked the variant names I gave this enum from the beginning and then I found out about rustfmt's `imports_granularity` config:
> imports_granularity
>
> How imports should be grouped into use statements. Imports will be merged or split to the configured level of granularity.
>
> Default value: Preserve
> Possible values: Preserve, Crate, Module, Item
> Stable: No
I personally prefer using `crate` over `full` and `module` over last, they seem more descriptive. Keeping these similar between tooling also seems like a good plus point to me.
We might even wanna take over the entire enum at some point if we have a `format/cleanup imports` assists in the future which would probably want to also have the `preserve` and `item` options.
Co-authored-by: Lukas Wirth <lukastw97@gmail.com>
There's a tension between keeping a well-architectured minimal
orthogonal set of constructs, and providing convenience functions.
Relieve this pressure by introducing an dedicated module for
non-orthogonal shortcuts.
This is inspired by the django.shortcuts module which serves a similar
purpose architecturally.
8776: fix: fix unnecessary recomputations due to macros r=jonas-schievink a=jonas-schievink
This computes a macro's fragment kind eagerly (when the calling file is still available in parsed form) and stores it in the `MacroCallLoc`. This means that during expansion we no longer have to reparse the file containing the macro call, avoiding the unnecessary salsa dependencies (https://github.com/rust-analyzer/rust-analyzer/pull/8746#issuecomment-834776349).
Marking as draft until I manage to find a test for this problem, since for some reason `typing_inside_a_function_should_not_invalidate_expansions` does not catch this (which might indicate that I misunderstand the problem).
I've manually confirmed that this fixes the issue described in https://github.com/rust-analyzer/rust-analyzer/pull/8746#issuecomment-834776349:
```
7ms - parse_query @ FileId(179)
12ms - SourceBinder::to_module_def
12ms - crate_def_map:wait
5ms - item_tree_query (1 calls)
7ms - ???
```
Co-authored-by: Jonas Schievink <jonasschievink@gmail.com>
8777: Escape characters in builtin macros correctly r=edwin0cheng a=edwin0cheng
Fixes#8749
It is the same bug in #8560 but in our `quote!` macro.
Because the "\" are adding exponentially in #8749 case, so the text is eat up all the memory.
bors r+
Co-authored-by: Edwin Cheng <edwin0cheng@gmail.com>
8774: feat: Honor `.cargo/config.toml` r=matklad a=Veykril
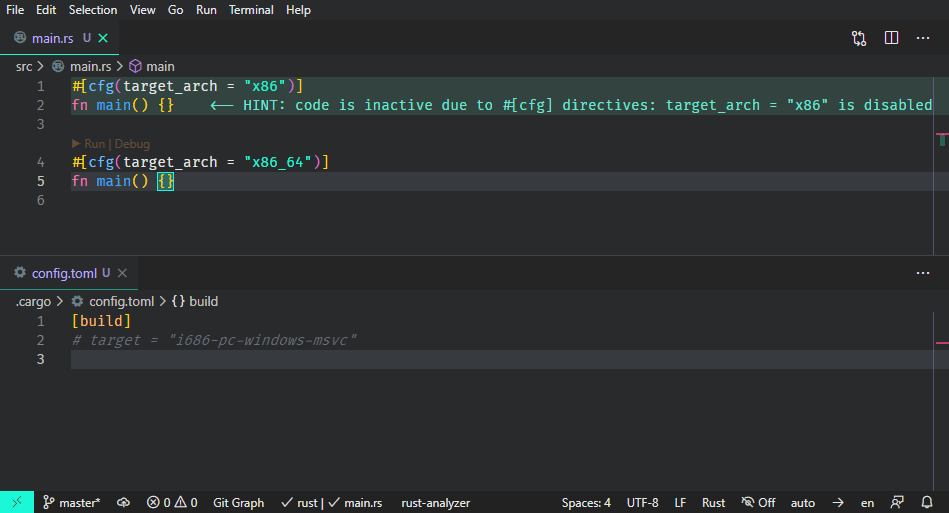
Implements `cargo/.config` build target and cfg access by using unstable cargo options:
- `cargo config get` to read the target triple out of the config to pass to `cargo metadata` --filter-platform
- `cargo rustc --print` to read out the `rustc_cfgs`, this causes us to honor `rustflags` and the like.
If those commands fail, due to not having a nightly toolchain present for example, they will fall back to invoking rustc directly as we currently do.
I personally think it should be fine to use these unstable options as they are unlikely to change(even if they did it shouldn't be a problem due to the fallback) and don't burden the user if they do not have a nightly toolchain at hand since we fall back to the previous behaviour.
cc #8741Closes#6604, Closes#5904, Closes#8430, Closes#8480
Co-authored-by: Lukas Wirth <lukastw97@gmail.com>
8745: Support goto_type_definition for types r=matklad a=Veykril
I'm unsure if the approach of lowering an `ast::Type` to a `hir::Type` is a good idea, it seems fine to me at least.
Fixes#2882
Co-authored-by: Lukas Tobias Wirth <lukastw97@gmail.com>
8280: Borrow text of immutable syntax node r=iDawer a=iDawer
In https://github.com/rust-analyzer/rowan/pull/101 `rowan::SyntaxNode::green` returns `Cow<'_, GreenNodeData>`. It returns borrow of green node of immutable syntax tree node.
Using this we can return borrowed text from `ast::Name::text`.
~~However now it allocates in case of mutable syntax trees.~~ (see next comment)
The idea comes from https://github.com/rust-analyzer/rowan/pull/100#issuecomment-809330325
Co-authored-by: Dawer <7803845+iDawer@users.noreply.github.com>
8674: fix for #8664: Emit folding ranges for multi-line where clauses r=matklad a=m5tfi
#8664
I added a test that assert folding multi-line where clauses while leaving single lined one. Please, let me know if the code needs further improvements.
Co-authored-by: m5tfi <72708423+m5tfi@users.noreply.github.com>
8711: Only resolve selected assist r=matklad a=SomeoneToIgnore
Part of https://github.com/rust-analyzer/rust-analyzer/issues/8700
Now resolves only the assist that was selected out of the list, while before the whole assist list was resolved despite a single popup selection.
Co-authored-by: Kirill Bulatov <mail4score@gmail.com>
8693: Ensure that only one cache priming task can run at a time r=matklad a=Bobo1239
Fixes#8632.
Co-authored-by: Boris-Chengbiao Zhou <bobo1239@web.de>
8692: Fix panic caused by new Try trait definition r=flodiebold a=flodiebold
The new Try trait definition caused a query cycle for us. This adds recovery for that cycle, but also fixes the cause, which is that we went through the supertraits when resolving `<T as Trait>::Assoc`, which isn't actually necessary. I also rewrote `all_super_trait_refs` to an iterator before I realized what the actual problem was, so I kept that.
Fixes#8686.
Co-authored-by: Florian Diebold <flodiebold@gmail.com>
According to the spec we should return ServerNotInitialized if the server is waiting for an initialize request and something else comes in.
Upgrading to lsp-server 0.5.1 will do this and retry until the initialize request comes in.
Fixes#8581
At the moment,the popup is just a bazillion of Cargo's "Compiling this\nCompiling that",
which is not that useful.
--quiet still displays error, which is what we needc
Attempting to rename an element of a tuple field would previously
replace the type with the new name, which doesn't make sense; now it
fails instead.
The check is done in both `prepare_rename` and `rename` so that the case
is caught before the user is prompted for a new name. Some other
existing failure cases are also now additionally checked in
`prepare_rename`.
8591: Remove SyntaxRewriter usage in insert_use in favor of mutable syntax trees r=matklad a=Veykril
Unfortunately changing `insert_use` to not use `SyntaxRewriter` creates a lot of changes since so much relies on that. But on the other hand this should be the biggest usage of `SyntaxRewriter` I believe.
8638: Remove SyntaxRewriter::from_fn r=Veykril a=Veykril
Co-authored-by: Lukas Wirth <lukastw97@gmail.com>
8620: Remove unnecessary braces for extracted block expression r=Veykril a=brandondong
This change addresses the first bullet point of https://github.com/rust-analyzer/rust-analyzer/issues/7839.
Specifically, when extracting block expressions, remove the unneeded extra braces inside the generated function.
Co-authored-by: Brandon <brandondong604@hotmail.com>
8570: Flycheck tries to parse both Cargo and Rustc messages. r=rickvanprim a=rickvanprim
This change allows non-Cargo build systems to be used for Flycheck provided they call `rustc` with `--error-format=json` and emit those JSON messages to `stdout`.
Co-authored-by: James Leitch <rickvanprim@gmail.com>
reading both stdout & stderr is a common gotcha, you need to drain them
concurrently to avoid deadlocks. Not sure why I didn't do the right
thing from the start. Seems like I assumed the stderr is short? That's
not the case when cargo spams `compiling xyz` messages
8524: Fix extract function with partial block selection r=matklad a=brandondong
**Reproduction:**
```rust
fn foo() {
let n = 1;
let mut v = $0n * n;$0
v += 1;
}
```
1. Select the snippet ($0) and use the "Extract into function" assist.
2. Extracted function is incorrect and does not compile:
```rust
fn foo() {
let n = 1;
let mut v = fun_name(n);
v += 1;
}
fn fun_name(n: i32) {}
```
3. Omitting the ending semicolon from the selection fixes the extracted function:
```rust
fn fun_name(n: i32) -> i32 {
n * n
}
```
**Cause:**
- When `extraction_target` uses a block extraction (semicolon case) instead of an expression extraction (no semicolon case), the user selection is directly used as the TextRange.
- However, the existing function extraction logic for blocks requires that the TextRange spans from start to end of complete statements to work correctly.
- For example:
```rust
fn foo() {
let m = 2;
let n = 1;
let mut v = m $0* n;
let mut w = 3;$0
v += 1;
w += 1;
}
```
produces
```rust
fn foo() {
let m = 2;
let n = 1;
let mut v = m let mut w = fun_name(n);
v += 1;
w += 1;
}
fn fun_name(n: i32) -> i32 {
let mut w = 3;
w
}
```
- The user selected TextRange is directly replaced by the function call which is now in the middle of another statement. The extracted function body only contains statements that were fully covered by the TextRange and so the `* n` code is deleted. The logic for calculating variable usage and outlived variables for the function parameters and return type respectively search within the TextRange and so do not include `m` or `v`.
**Fix:**
- Only extract full statements when using block extraction. If a user selected part of a statement, extract that full statement.
8527: Switch introduce_named_lifetime assist to use mutable syntax tree r=matklad a=iDawer
This extends `GenericParamsOwnerEdit` trait with `get_or_create_generic_param_list` method
Co-authored-by: Brandon <brandondong604@hotmail.com>
Co-authored-by: Dawer <7803845+iDawer@users.noreply.github.com>