Inline all format arguments where possible
This makes code more readale and concise,
moving all format arguments like `format!("{}", foo)` into the more compact `format!("{foo}")` form.
The change was automatically created with, so there are far less change of an accidental typo.
```
cargo clippy --fix -- -A clippy::all -W clippy::uninlined_format_args
```
This makes code more readale and concise,
moving all format arguments like `format!("{}", foo)`
into the more compact `format!("{foo}")` form.
The change was automatically created with, so there are far less change
of an accidental typo.
```
cargo clippy --fix -- -A clippy::all -W clippy::uninlined_format_args
```
Clippy-fix explicit auto-deref
Seems like these can be safely fixed. With one, I was particularly surprised -- `Some(pats) => &**pats,` in body.rs?
```
cargo clippy --fix -- -A clippy::all -D clippy::explicit_auto_deref
```
Seems like these can be safely fixed. With one, I was particularly
surprised -- `Some(pats) => &**pats,` in body.rs?
```
cargo clippy --fix -- -A clippy::all -D clippy::explicit_auto_deref
```
Remove non-needed clones
I am not certain if this will improve performance, but it seems having a .clone() without any need should be removed.
This was done with clippy, and manually reviewed:
```
cargo clippy --fix -- -A clippy::all -D clippy::redundant_clone
```
I am not certain if this will improve performance,
but it seems having a .clone() without any need should be removed.
This was done with clippy, and manually reviewed:
```
cargo clippy --fix -- -A clippy::all -D clippy::redundant_clone
```
feat: Add an option to hide adjustment hints outside of `unsafe` blocks and functions
As the title suggests: this PR adds an option (namely `rust-analyzer.inlayHints.expressionAdjustmentHints.hideOutsideUnsafe`) that allows to hide adjustment hints outside of `unsafe` blocks and functions:
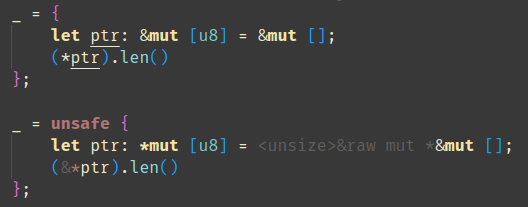
Requested by `@BoxyUwU` <3
fix: Correctly check for parentheses redundancy in `remove_parentheses` assist
This is quite a bunch of code and some hacks, but I _think_ this time it's correct.
I've added a lot of tests, most of which fail with the assist impl from #13733 :')
Complete enum variants without parens when snippets are disabled
This handles the portion of #13767 that bothered me, but I can try to work on the other parts we discussed if needed.
fix: Use the correct edition when formatting code in path dependencies
Fixes https://github.com/rust-lang/rust-analyzer/issues/13790
Don't go through the Cargo workspace info, since that doesn't contain path dependencies. Instead, query the crate graph via `Analysis::crate_edition`.
fix: fix "parser seems stuck" panic when parsing colossal files
The parser step count is incremented every time the parser inspects a token. It's purpose is to ensure the parser doesn't get stuck in infinite loops. But since `self.pos` grows monotonically when parsing source code, it gives a better idea for whether the parser is stuck or not: if `self.pos` is changed, we know that the parser cannot be stuck, so it is safe to reset the step count to 0. This makes the limit check scale with the size of the file, and so should fix https://github.com/rust-lang/rust-analyzer/issues/13788.