Prevent fmt::Arguments from being shared across threads
Fixes#45197
This is a **breaking change**! Without doing this it's very easy to create race conditions.
There's probably a way to do this without breaking valid use cases, but it would require quite an overhaul of the formatting machinery.
rustdoc: include external files in documentation (RFC 1990)
Part of https://github.com/rust-lang/rfcs/pull/1990 (needs work on the error reporting, which i'm deferring to after this initial PR)
cc #44732
Also fixes#42760, because the prep work for the error reporting made it easy to fix that at the same time.
MIR-borrowck: Some minor fixes
- Remove parens when printing dereference (fix#45185)
- Change argument type of `autoderef` to `bool`
- Change argument type of `field_index` to `Field`
move closure kind, signature into `ClosureSubsts`
Instead of using side-tables, store the closure-kind and signature in the substitutions themselves. This has two key effects:
- It means that the closure's type changes as inference finds out more things, which is very nice.
- As a result, it avoids the need for the `freshen_closure_like` code (though we still use it for generators).
- It avoids cyclic closures calls.
- These were never meant to be supported, precisely because they make a lot of the fancy inference that we do much more complicated. However, due to an oversight, it was previously possible -- if challenging -- to create a setup where a closure *directly* called itself (see e.g. #21410).
We have to see what the effect of this change is, though. Needs a crater run. Marking as [WIP] until that has been assessed.
r? @arielb1
clean the Debug impl for CrateNum and DefId
Just a tiny quality-of-life improvement because I got tired of noisy debug logs.
```
before: DefId { krate: CrateNum(11), index: DefIndex(0:6) => foo[8787]::Mapper[0]::OtherType[0] } }
after: {crate11:0:6 ~ foo[8787]::Mapper[0]::OtherType[0]})
```
r? @michaelwoerister
dead code lint to say "never constructed" for variants
As reported in #19140, #44083, and #44565, some users were confused when
the dead-code lint reported an enum variant to be "unused" when it was
matched on (but not constructed). This wording change makes it clearer
that the lint is in fact checking for construction.
We continue to say "used" for all other items (it's tempting to say
"called" for functions and methods, but this turns out not to be
correct: functions can be passed as arguments and the dead-code lint
isn't special-casing that or anything).
Resolves#19140.
r? @pnkfelix
Optimize `read_to_end`.
This patch makes `read_to_end` use Vec's memory-growth pattern rather
than using a custom pattern.
This has some interesting effects:
- If memory is reserved up front, `read_to_end` can be faster, as it
starts reading at the buffer size, rather than always starting at 32
bytes. This speeds up file reading by 2x in one of my use cases.
- It can reduce the number of syscalls when reading large files.
Previously, `read_to_end` would settle into a sequence of 8192-byte
reads. With this patch, the read size follows Vec's allocation
pattern. For example, on a 16MiB file, it can do 21 read syscalls
instead of 2057. In simple benchmarks of large files though, overall
speed is still dominated by the actual I/O.
- A downside is that Read implementations that don't implement
`initializer()` may see increased memory zeroing overhead.
I benchmarked this on a variety of data sizes, with and without
preallocated buffers. Most benchmarks see no difference, but reading
a small/medium file with a pre-allocated buffer is faster.
rustbuild: distribute cargo-fmt alongside rustfmt
Not sure whether we want that nor if it's the right way to do so, but it feels quite weird to have rustfmt without cargo-fmt. Or are there other plans wrt that?
What do you think @nrc ?
update let-expressions hash test to use `except`
A part of #44924, this PR updated let-expressions test using `except`.
cc @michaelwoerister
r? @nikomatsakis
impl Trait Lifetime Handling
This PR implements the updated strategy for handling `impl Trait` lifetimes, as described in [RFC 1951](https://github.com/rust-lang/rfcs/blob/master/text/1951-expand-impl-trait.md) (cc #42183).
With this PR, the `impl Trait` desugaring works as follows:
```rust
fn foo<T, 'a, 'b, 'c>(...) -> impl Foo<'a, 'b> { ... }
// desugars to
exists type MyFoo<ParentT, 'parent_a, 'parent_b, 'parent_c, 'a, 'b>: Foo<'a, 'b>;
fn foo<T, 'a, 'b, 'c>(...) -> MyFoo<T, 'static, 'static, 'static, 'a, 'b> { ... }
```
All of the in-scope (parent) generics are listed as parent generics of the anonymous type, with parent regions being replaced by `'static`. Parent regions referenced in the `impl Trait` return type are duplicated into the anonymous type's generics and mapped appropriately.
One case came up that wasn't specified in the RFC: it's possible to write a return type that contains multiple regions, neither of which outlives the other. In that case, it's not clear what the required lifetime of the output type should be, so we generate an error.
There's one remaining FIXME in one of the tests: `-> impl Foo<'a, 'b> + 'c` should be able to outlive both `'a` and `'b`, but not `'c`. Currently, it can't outlive any of them. @nikomatsakis and I have discussed this, and there are some complex interactions here if we ever allow `impl<'a, 'b> SomeTrait for AnonType<'a, 'b> { ... }`, so the plan is to hold off on this until we've got a better idea of what the interactions are here.
cc #34511.
Fixes#44727.
show macro backtrace with -Z flag
Fixes#39413 by adding a facility to restore the "old school" macro expansion backtraces (previously removed in 61865384b8).
The restored functionality is accessed through the flag `-Z external-macro-backtrace`. Errors showing the truncated backtraces will suggest this flag.
### Example
Code: <details>
`a/src/lib.rs`
```rust
#[macro_export]
macro_rules! a {
() => { a!(@) };
(@) => { a!(@@) };
(@@) => {
syntax error;
}
}
```
`b/src/main.rs`
```rust
#[macro_use] extern crate a;
macro_rules! b {
() => { b!(@) };
(@) => { b!(@@) };
(@@) => {
syntax error;
}
}
fn main() {
a!();
b!();
}
```
</details>
<br/><br/>
Running without env var (note: first error is from remote macro, second from local macro):
<details>
```
$ cargo +custom run
Compiling b v0.1.0
error: expected one of `!`, `.`, `::`, `;`, `?`, `{`, `}`, or an operator, found `error`
--> src/main.rs:12:5
|
12 | a!();
| ^^^^^
| |
| expected one of 8 possible tokens here
| unexpected token
|
= note: this error originates in a macro outside of the current crate (run with RUST_MACRO_BACKTRACE=1 for more info)
error: expected one of `!`, `.`, `::`, `;`, `?`, `{`, `}`, or an operator, found `error`
--> src/main.rs:7:16
|
7 | syntax error;
| -^^^^^ unexpected token
| |
| expected one of 8 possible tokens here
...
13 | b!();
| ----- in this macro invocation
error: aborting due to 2 previous errors
error: Could not compile `b`.
To learn more, run the command again with --verbose.
```
</details>
The output is the same as today, except for an addition to the note which aids discoverability of the new environment variable.
<br/><br/>
Running _with_ env var:
<details>
```
$ RUST_MACRO_BACKTRACE=1 cargo +custom run
Compiling b v0.1.0
error: expected one of `!`, `.`, `::`, `;`, `?`, `{`, `}`, or an operator, found `error`
--> <a macros>:1:72
|
1 | ( ) => { a ! ( @ ) } ; ( @ ) => { a ! ( @ @ ) } ; ( @ @ ) => { syntax error ;
| -^^^^^ unexpected token
| |
| expected one of 8 possible tokens here
src/main.rs:12:5: 12:10 note: in this expansion of a! (defined in <a macros>)
<a macros>:1:11: 1:20 note: in this expansion of a! (defined in <a macros>)
<a macros>:1:36: 1:47 note: in this expansion of a! (defined in <a macros>)
error: expected one of `!`, `.`, `::`, `;`, `?`, `{`, `}`, or an operator, found `error`
--> src/main.rs:7:16
|
7 | syntax error;
| -^^^^^ unexpected token
| |
| expected one of 8 possible tokens here
src/main.rs:12:5: 12:10 note: in this expansion of a! (defined in <a macros>)
<a macros>:1:11: 1:20 note: in this expansion of a! (defined in <a macros>)
<a macros>:1:36: 1:47 note: in this expansion of a! (defined in <a macros>)
error: expected one of `!`, `.`, `::`, `;`, `?`, `{`, `}`, or an operator, found `error`
--> src/main.rs:7:16
|
7 | syntax error;
| -^^^^^ unexpected token
| |
| expected one of 8 possible tokens here
src/main.rs:13:5: 13:10 note: in this expansion of b! (defined in src/main.rs)
src/main.rs:4:13: 4:18 note: in this expansion of b! (defined in src/main.rs)
src/main.rs:5:14: 5:20 note: in this expansion of b! (defined in src/main.rs)
error: aborting due to 2 previous errors
error: Could not compile `b`.
To learn more, run the command again with --verbose.
```
</details>
The output is hard to read, but better than nothing (and it's exactly what we used to have before the infamous `fix_multispans_in_std_macros`).
<br/><br/>
Wishlist:
- Save the actual source of macros in crate metadata, not just AST, so the output can be improved
- Hopefully this would allow line numbers in the trace as well
- Show the actual macro invocations in the traces
r? @nrc
show in docs whether the return type of a function impls Iterator/Read/Write
Closes#25928
This PR makes it so that when rustdoc documents a function, it checks the return type to see whether it implements a handful of specific traits. If so, it will print the impl and any associated types. Rather than doing this via a whitelist within rustdoc, i chose to do this by a new `#[doc]` attribute parameter, so things like `Future` could tap into this if desired.
### Known shortcomings
~~The printing of impls currently uses the `where` class over the whole thing to shrink the font size relative to the function definition itself. Naturally, when the impl has a where clause of its own, it gets shrunken even further:~~ (This is no longer a problem because the design changed and rendered this concern moot.)
The lookup currently just looks at the top-level type, not looking inside things like Result or Option, which renders the spotlights on Read/Write a little less useful:
<details><summary>`File::{open, create}` don't have spotlight info (pic of old design)</summary>
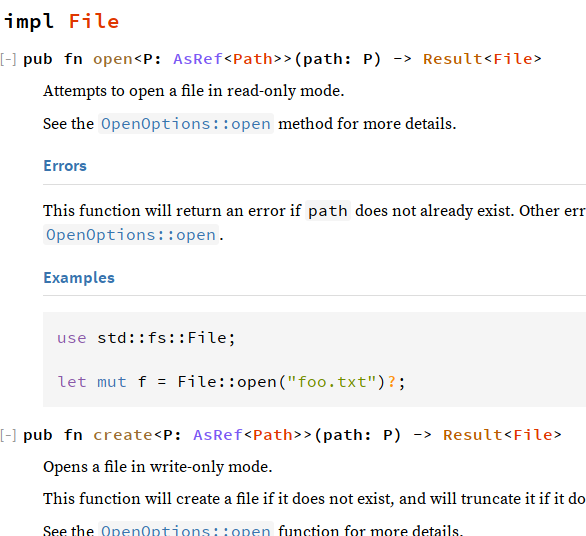
</details>
All three of the initially spotlighted traits are generically implemented on `&mut` references. Rustdoc currently treats a `&mut T` reference-to-a-generic as an impl on the reference primitive itself. `&mut Self` counts as a generic in the eyes of rustdoc. All this combines to create this lovely scene on `Iterator::by_ref`:
<details><summary>`Iterator::by_ref` spotlights Iterator, Read, and Write (pic of old design)</summary>
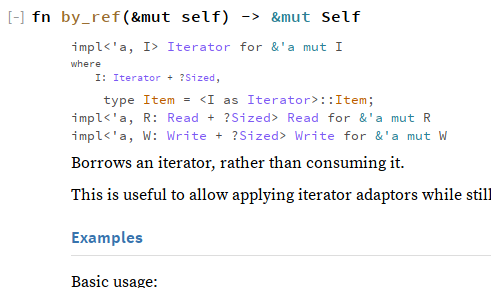
</details>
[rustllvm] Use report_fatal_error over llvm_unreachable
This makes it more robust when assertions are disabled, crashing instead of causing UB.
Also introduces a tidy check to enforce this rule, which in turn necessitated making tidy run on `src/rustllvm`.
Fixes#44020
Fix typo in MIR "cannot move out of borrowed content"
I believe this all we need to change (#46018). Anyway, do let me know if there is anything else that needs to changed as well!
add doc for doing `Read` from `&str`
This information can be found on [stackoverflow](https://stackoverflow.com/questions/32674905/pass-string-to-function-taking-read-trait) but I think it would be beneficial if it was documented in the `Read` trait itself.
I had an *extremely* hard time finding this information, and "mocking" a reader with a string is an EXTREMELY common thing (I believe).
impl From for Mutex and RwLock
I felt that these implementations were missing, because doing `x.into()` works for other smart containers (such as `RefCell`), and in general I would say that the conversion makes sense.