Fix failing codegen tests on s390x
Several codegen tests are currently failing due to making assumptions that are not valid for the s390x architecture:
- catch-unwind.rs: fails due to inlining differences. Already ignored on another platform for the same reason. Solution: Ignore on s390x.
- remap_path_prefix/main.rs: fails due to different alignment requirement for string constants. Solution: Do not test for the alignment requirement.
- repr-transparent-aggregates-1.rs: many ABI assumptions. Already ignored on many platforms for the same reason. Solution: Ignore on s390x.
- repr-transparent.rs: no vector ABI by default on s390x. Already ignored on another platform for a similar reason. Solution: Ignore on s390x.
- uninit-consts.rs: hard-coded little-endian constant. Solution: Match both little- and big-endian versions.
Fixes part of https://github.com/rust-lang/rust/issues/105383.
Rollup of 10 pull requests
Successful merges:
- #98391 (Reimplement std's thread parker on top of events on SGX)
- #104019 (Compute generator sizes with `-Zprint_type_sizes`)
- #104512 (Set `download-ci-llvm = "if-available"` by default when `channel = dev`)
- #104901 (Implement masking in FileType comparison on Unix)
- #105082 (Fix Async Generator ABI)
- #105109 (Add LLVM KCFI support to the Rust compiler)
- #105505 (Don't warn about unused parens when they are used by yeet expr)
- #105514 (Introduce `Span::is_visible`)
- #105516 (Update cargo)
- #105522 (Remove wrong note for short circuiting operators)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
Remove wrong note for short circuiting operators
They *are* representable by traits, even if the short-circuiting behaviour requires a different approach than the non-short-circuiting operators. For an example proposal, see the postponed [RFC 2722](https://github.com/rust-lang/rfcs/pull/2722). As it is not accurate, remove most of the note.
Add LLVM KCFI support to the Rust compiler
This PR adds LLVM Kernel Control Flow Integrity (KCFI) support to the Rust compiler. It initially provides forward-edge control flow protection for operating systems kernels for Rust-compiled code only by aggregating function pointers in groups identified by their return and parameter types. (See llvm/llvm-project@cff5bef.)
Forward-edge control flow protection for C or C++ and Rust -compiled code "mixed binaries" (i.e., for when C or C++ and Rust -compiled code share the same virtual address space) will be provided in later work as part of this project by identifying C char and integer type uses at the time types are encoded (see Type metadata in the design document in the tracking issue #89653).
LLVM KCFI can be enabled with -Zsanitizer=kcfi.
Thank you again, `@bjorn3,` `@eddyb,` `@nagisa,` and `@ojeda,` for all the help!
Fix Async Generator ABI
This change was missed when making async generators implement `Future` directly.
It did not cause any problems in codegen so far, as `GeneratorState<(), Output>`
happens to have the same ABI as `Poll<Output>`.
Set `download-ci-llvm = "if-available"` by default when `channel = dev`
See https://github.com/rust-lang/compiler-team/issues/566. The motivation for changing the default is to avoid downloading and building LLVM when someone runs `x build` before running `x setup`. The motivation for only doing it on `channel = "dev"` is to avoid breaking distros or users installing from source. It works because `dev` is also the default channel.
The diff looks larger than it is; most of it is moving the `llvm` branch below the `rust` so `config.channel` is set.
r? `@Mark-Simulacrum` cc `@oli-obk` `@bjorn3` `@cuviper`
Reimplement std's thread parker on top of events on SGX
Mutex and Condvar are being replaced by more efficient implementations, which need thread parking themselves (see #93740). Therefore, the generic `Parker` needs to be replaced on all platforms where the new lock implementation will be used.
SGX enclaves have a per-thread event state, which allows waiting for and setting specific bits. This is already used by the current mutex implementation. The thread parker can however be much more efficient, as it only needs to store the `TCS` address of one thread. This address is stored in a state variable, which can also be set to indicate the thread was already notified.
`park_timeout` does not guard against spurious wakeups like the current condition variable does. This is allowed by the API of `Parker`, and I think it is better to let users handle these wakeups themselves as the guarding is quite expensive and might not be necessary.
`@jethrogb` as you wrote the initial SGX support for `std`, I assume you are the target maintainer? Could you help me test this, please? Lacking a x86_64 chip, I can't run SGX.
They *are* representable by traits, even if the short-circuiting
behaviour requires a different approach than the non-short-circuiting
operators. For an example proposal, see the postponed RFC 2722.
As it is not accurate, reword the note.
Rollup of 9 pull requests
Successful merges:
- #102406 (Make `missing_copy_implementations` more cautious)
- #105265 (Add `rustc_on_unimplemented` to `Sum` and `Product` trait.)
- #105385 (Skip test on s390x as LLD does not support the platform)
- #105453 (Make `VecDeque::from_iter` O(1) from `vec(_deque)::IntoIter`)
- #105468 (Mangle "main" as "__main_void" on wasm32-wasi)
- #105480 (rustdoc: remove no-op mobile CSS `#sidebar-toggle { text-align }`)
- #105489 (Fix typo in apple_base.rs)
- #105504 (rustdoc: make stability badge CSS more consistent)
- #105506 (Tweak `rustc_must_implement_one_of` diagnostic output)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
rustdoc: remove no-op mobile CSS `#sidebar-toggle { text-align }`
Since 8b001b4da0716936e0ca32303cc0e3c5e53e42f8 make the sidebar toggle a flex container, and already centers its content in desktop mode, this rule doesn't do anything.
Mangle "main" as "__main_void" on wasm32-wasi
On wasm, the age-old C trick of having a main function which can either have no arguments or argc+argv doesn't work, because wasm requires caller and callee signatures to match. WASI's current strategy is to have compilers mangle main's name to indicate which signature they're using. Rust uses the no-argument form, which should be mangled as `__main_void`.
This is needed on wasm32-wasi as of #105395.
Make `VecDeque::from_iter` O(1) from `vec(_deque)::IntoIter`
As suggested in https://github.com/rust-lang/rust/pull/105046#issuecomment-1330371695 by
r? ``@the8472``
`Vec` & `VecDeque`'s `IntoIter`s own the allocations, and even if advanced can be turned into `VecDeque`s in O(1).
This is just a specialization, not an API or doc commitment, so I don't think it needs an FCP.
Skip test on s390x as LLD does not support the platform
test/run-make/issue-71519 requires use of lld as linker, but lld does not currently support the s390x architecture.
Make `missing_copy_implementations` more cautious
- Fixes https://github.com/rust-lang/rust/issues/98348
- Also makes the lint not fire on large types and types containing raw pointers. Thoughts?
Shrink `rustc_parse_format::Piece`
This makes both variants closer together in size (previously they were different by 208 bytes -- 16 vs 224). This may make things worse, but it's worth a try.
r? `@nnethercote`
* They all get rounded corners now. A test case has been added for this, too.
* There are now broadly two kinds of stability badge, where there used to be
three: item-info "fat badge", and the "thin badge" in both item tables and
in docblocks (which got merged). The fat badges can have icons, while the
thin badges can't.
Clippy: backport ICE fix before beta branch
r? `@Manishearth`
Before beta is branched tomorrow we should backport the fix from https://github.com/rust-lang/rust-clippy/pull/10027 for an ICE. That way we'll get this into stable one release sooner.
This only cherry-picks the fix, not the tests for it. The proper sync of this will be done next week Thursday.
use the correct `Reveal` during validation
supersedes #105454. Deals with https://github.com/rust-lang/rust/issues/105009#issuecomment-1342395333, not closing #105009 as the ICE may leak into beta
The issue was the following:
- we optimize the mir, using `Reveal::All`
- some optimization relies on the hidden type of an opaque type
- we then validate using `Reveal::UserFacing` again which is not able to observe the hidden type
r? `@jackh726`
Move some queries and methods
Each commit's title should be self-explanatory. Motivated to break up some large, general files and move queries into leaf crates.
Dont silently ignore rustdoc errors
I applied the suggestions from https://github.com/rust-lang/rust/pull/104995 and also checked the rustdoc-ui error but couldn't reproduce it.
r? `@notriddle`
Enable profiler in dist-powerpc64le-linux
Build the profiler runtime to allow using -C profile-generate and -C instrument-coverage on POWER little endian systems.
I have verified locally that the runtime builds and the profiler is working fine on the platform.
Similar pull request for a different system: https://github.com/rust-lang/rust/pull/104304
Improve Rustdoc scrape-examples UI
This PR combines a few different improvements to the scrape-examples UI. See a live demo here: https://willcrichton.net/misc/scrape-examples/small-first-example/clap/struct.Arg.html
### 1. The first scraped example now takes up significantly less screen height.
Inserting the first scraped example takes up a lot of vertical screen space. I don't want this addition to overwhelm users, so I decided to reduce the height of the initial example in two ways: (A) the default un-expanded height is reduced from 240px (10 LOC) to 120px (5 LOC), and (B) the link to the example is now positioned *over* the example instead of *atop* the example (only on desktop though, not mobile). The changes to `scrape-examples.js` and `rustdoc.css` implement this fix.
Here is what an example docblock now looks like:
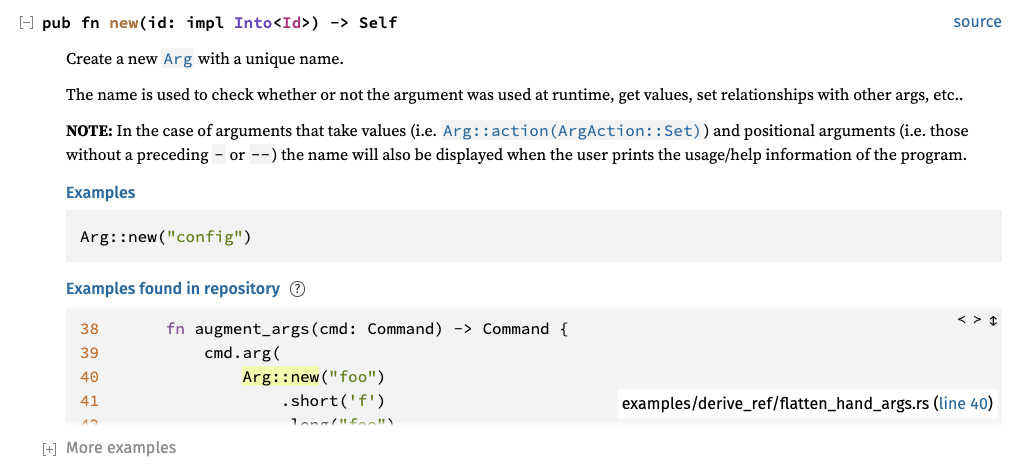
### 2. Expanding all docblocks will not expand "More examples".
The "More examples blocks" are huge, so fully expanding everything on the page would take up too much vertical space. The changes to `main.js` implement this fix. This is tested in `scrape-examples-toggle.goml`.
### 3. Examples from binary crates are sorted higher than examples from library crates.
Code that is written as an example of an API is probably better for learning than code that happens to use an API, but isn't intended for pedagogic purposes. Unfortunately Rustc doesn't know whether a particular crate comes from an example target (only Cargo knows this). But we can at least create a proxy that prefers examples from binary crates over library crates, which we know from `--crate-type`.
This change is implemented by adding a new field `bin_crate` in `Options` (see `config.rs`). An `is_bin` field has been added to the scraped examples metadata (see `scrape_examples.rs`). Then the example sorting metric uses `is_bin` as the first entry of a lexicographic sort on `(is_bin, example_size, display_name)` (see `render/mod.rs`).
Note that in the future we can consider adding another flag like `--scrape-examples-cargo-target` that would pass target information from Cargo into the example metadata. But I'm proposing a less intrusive change for now.
### 4. The scrape-examples help page has been updated to reflect the latest Cargo interface.
See `scrape-examples-help.md`.
r? `@notriddle`
P.S. once this PR and rust-lang/cargo#11450 are merged, then I think the scrape-examples feature is officially ready for deployment on docs.rs!