The proper attribute was added to `simd_shuffle*` in
rust-lang/stdarch#825. This caused `promote_consts` to double-count its
second argument when recording promotion candidates, which caused
the promotion candidate compatibility check to fail.
Partially revert the early feature-gatings added in #65742.
The intent here is to address #65860 ASAP (in time for beta, ideally), while leaving as much of #65742 around as possible, to make it easier to re-enable later.
Therefore, I've only kept the parts of the revert that re-add the old (i.e. non-early) feature-gating checks that were removed in #65742, and the test reverts.
I've disabled the new early feature-gating checks from #65742 entirely for now, but it would be easy to put them behind a `-Z` flag, or turn them into warnings, which would allow us to keep tests for both the early and late versions of the checks - assuming that's desirable.
cc @nikomatsakis @Mark-Simulacrum @Centril
Rollup of 16 pull requests
Successful merges:
- #65112 (Add lint and tests for unnecessary parens around types)
- #65470 (Don't hide ICEs from previous incremental compiles)
- #65471 (Add long error explanation for E0578)
- #65857 (rustdoc: Resolve module-level doc references more locally)
- #65902 (Make ItemContext available for better diagnositcs)
- #65914 (Use structured suggestion for unnecessary bounds in type aliases)
- #65946 (Make `promote_consts` emit the errors when required promotion fails)
- #65960 (doc: reword iter module example and mention other methods)
- #65963 (update submodules to rust-lang)
- #65972 (Fix libunwind build: Define __LITTLE_ENDIAN__ for LE targets)
- #65977 (Fix incorrect diagnostics for expected type in E0271 with an associated type)
- #65995 (Add error code E0743 for "C-variadic has been used on a non-foreign function")
- #65997 (Fix outdated rustdoc of Once::init_locking function)
- #66002 (Stabilize float_to_from_bytes feature)
- #66005 (vxWorks: remove code related unix socket)
- #66018 (Revert PR 64324: dylibs export generics again (for now))
Failed merges:
r? @ghost
Revert PR 64324: dylibs export generics again (for now)
As discussed on PR #65781, this is a targeted attempt to undo the main semantic change from PR #64324, by putting `dylib` back in the set of crate types that export generic symbols.
The main reason to do this is that PR #64324 had unanticipated side-effects that caused bugs like #64872, and in the opinion of @alexcrichton and myself, the impact of #64872 is worse than #64319.
In other words, it is better for us, in the short term, to reopen#64319 as currently unfixed for now than to introduce new bugs like #64872.
Fix#64872Reopen#64319
Fix incorrect diagnostics for expected type in E0271 with an associated type
With code like the following code:
```rust
#[derive(Debug)]
struct Data {}
fn do_stuff<'a>(iterator: impl Iterator<Item = &'a Data>) {
for item in iterator {
println!("{:?}", item)
}
}
fn main() {
let v = vec![Data {}];
do_stuff(v.into_iter());
}
```
the diagnostic (in nightly & stable) wrongly complains about the expected type:
```
error[E0271]: type mismatch resolving `<std::vec::IntoIter<Data> as std::iter::Iterator>::Item == &Data`
--> src/main.rs:15:5
|
5 | fn do_stuff<'a>(iterator: impl Iterator<Item = &'a Data>) {
| -------- --------------- required by this bound in `do_stuff`
...
15 | do_stuff(v.into_iter());
| ^^^^^^^^ expected struct `Data`, found &Data
|
= note: expected type `Data`
found type `&Data`
```
This PR fixes this issue by flipping the expected/actual values where appropriate, so it looks like this:
```
error[E0271]: type mismatch resolving `<std::vec::IntoIter<Data> as std::iter::Iterator>::Item == &Data`
--> main.rs:15:5
|
5 | fn do_stuff<'a>(iterator: impl Iterator<Item = &'a Data>) {
| -------- --------------- required by this bound in `do_stuff`
...
15 | do_stuff(v.into_iter());
| ^^^^^^^^ expected &Data, found struct `Data`
|
= note: expected type `&Data`
found type `Data`
```
This improves the output of a lot of existing tests (check out `associated-types-binding-to-type-defined-in-supertrait`!).
The only change which I wasn't too sure about is in the test `associated-types-overridden-binding-2`, but I think it's an improvement and the underlying problem is with handling of `trait_alias`.
Fix#57226, fix#64760, fix#58092.
Fix libunwind build: Define __LITTLE_ENDIAN__ for LE targets
If `__LITTLE_ENDIAN__` is missing, libunwind assumes big endian
and reads unwinding instructions wrong on ARM EHABI.
Fix#65765
Technical background in referenced bug.
I didn't run any automated tests, just built a simple panicking program using the fixed toolchain and panicking started to work. Tried with `catch_unwind()` and that seems to work now too. libunwind's log seems ok now, I can paste it if needed.
update submodules to rust-lang
These are the repositories I've moved from rust-lang-nursery to
rust-lang, so we should update the submodules too.
I have not tested that this builds at all, so please make sure CI is green!
Make `promote_consts` emit the errors when required promotion fails
A very minimal version of #65942.
This will cause a generic "argument X is required to be a constant" message for `simd_shuffle` LLVM intrinsics instead of the [custom one](caa1f8d7b3/src/librustc_mir/transform/qualify_consts.rs (L1616)). It may be possible to remove this special-casing altogether after rust-lang/stdarch#825.
r? @eddyb
rustdoc: Resolve module-level doc references more locally
Module level docs should resolve intra-doc links as locally as
possible. As such, this commit alters the heuristic for finding
intra-doc links such that we attempt to resolve names mentioned
in *inner* documentation comments within the (sub-)module rather
that from the context of its parent.
I'm hoping that this fixes#55364 though right now I'm not sure it's the right fix.
r? @GuillaumeGomez
Don't hide ICEs from previous incremental compiles
I think this fixes#65401, the compiler does not fail to ICE after the first compilation, tested on the last snippet of [this comment](https://github.com/rust-lang/rust/issues/63154#issuecomment-541592381).
I am not very sure of the fix as I don't understand much of the structure of the compiler.
Add lint and tests for unnecessary parens around types
This is my first contribution to the Rust project, so I apologize if I'm not doing things the right way.
The PR fixes#64169. It adds a lint and tests for unnecessary parentheses around types. I've run `tidy` and `rustfmt` — I'm not totally sure it worked right, though — and I've tried to follow the instructions linked in the readme.
I tried to think through all the variants of `ast::TyKind` to find exceptions to this lint, and I could only find the one mentioned in the original issue, which concerns types with `dyn`. I'm not a Rust expert, thought, so I may well be missing something.
There's also a problem with getting this to build. The new lint catches several things in the, e.g., `core`. Because `x.py` seems to build with an equivalent of `-Werror`, what would have been warnings cause the build to break. I got it to build and the tests to pass with `--warnings warn` on my `x.py build` and `x.py test` commands.
(Many thanks to alex for 1. making this even smaller than what I had
originally minimized, and 2. pointing out that there is precedent for
having ui tests with crate dependency chains of length > 2, thus
allowing me to avoid encoding this as a run-make test.)
rustc_codegen_ssa: introduce MIR VarDebugInfo, but only for codegen.
These are all the codegen changes necessary for #56231.
The refactors were performed locally to codegen, and in several steps, to ease reviewing and avoid introducing changes in behavior (as I'm not sure our debuginfo tests cover enough).
r? @michaelwoerister cc @nagisa @rkruppe @oli-obk
Dual proc macro hash
This PR changes current `-Z dual-proc-macro` mechanism from resolving only by name to including the hash of the host crate inside the transistive dependency information to prevent name conflicts.
Fix partially #62558
Fix `-Zunpretty=mir-cfg` to render multiple items
`-Zunpretty=mir-cfg` outputs DOT to stdout for all items being compiled. However, it puts all of these items in separate `digraph`s, which means the result of redirecting that output to a file is not valid. Most dot renderers (I have tried `dot` and `xdot`) cannot render the output.
This PR checks to see if `write_mir_graphviz` will process multiple items, and writes them each as a `subgraph` in a single, top-level `digraph`. As a result, DOT can be viewed without manually editing the output file. The output is unchanged when printing a single item (e.g.`-Zunpretty=mir-cfg=item_name`).
Here's the output of `xdot` for a rust file containing three items:
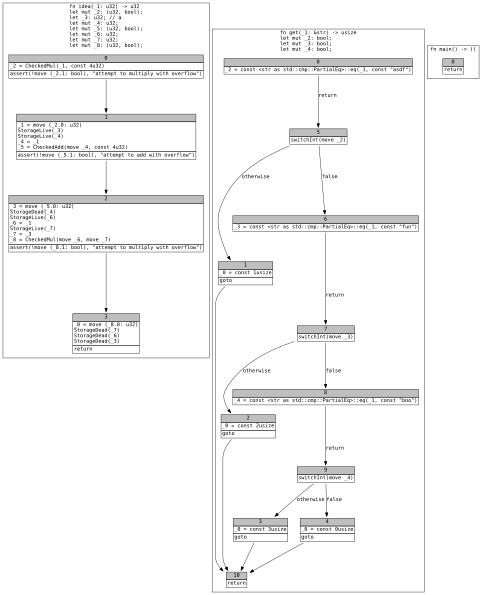
The borders are a result of the nonstandard–but well-supported–[`cluster` prefix](https://graphviz.gitlab.io/_pages/doc/info/lang.html) (search for "Subgraphs and Clusters"). They will not appear if your renderer does not support this extension, but the graph will still render properly.
Implement ordered/sorted iterators on BinaryHeap as per #59278
I've implemented the ordered version of iterator on BinaryHeap as per #59278.
# Added methods:
* `.into_iter_sorted()`
* like `.into_iter()`; but returns elements in heap order
* `.drain_sorted()`
* like `.drain()`; but returns elements in heap order
* It's a bit _lazy_; elements are removed on drop. (Edit: it’s similar to vec::Drain)
For `DrainSorted` struct, I implemented `Drop` trait following @scottmcm 's [suggestion](https://github.com/rust-lang/rust/issues/59278#issuecomment-537306925)
# ~TODO~ DONE
* ~I think I need to add more tests other than doctest.~
# **Notes:**
* we renamed `_ordered` to `_sorted`, because the latter is more common in rust libs. (as suggested by @KodrAus )
[rustdoc] stabilize cfg(doctest)
Fixes#62210.
Since we removed rustdoc from providing cfg(test) on test runs, it's been replaced by cfg(doctest). It'd be nice to have it in not too far in the future.