(The present author fears not being knowledgeable enough to rewrite the
comments unilaterally; merely calling it out is a lazy half-measure, but
at least doesn't actively make things worse the way an ill-informed
rewrite would.)
add modifier keyword spans to hir::Visibility; improve unreachable-pub, private-no-mangle lint suggestions
#50455 pointed out that the unreachable-pub suggestion for brace-grouped `use`s was bogus; #50476 partially ameliorated this by marking the suggestion as `Applicability::MaybeIncorrect`, but this is the actual fix.
Meanwhile, another application of having spans available in `hir::Visibility` is found in the private-no-mangle lints, where we can now issue a suggestion to use `pub` if the item has a more restricted visibility marker (this seems much less likely to come up in practice than not having any visibility keyword at all, but thoroughness is a virtue). While we're there, we can also add a helpful note if the item does have a `pub` (but triggered the lint presumably because enclosing modules were private).
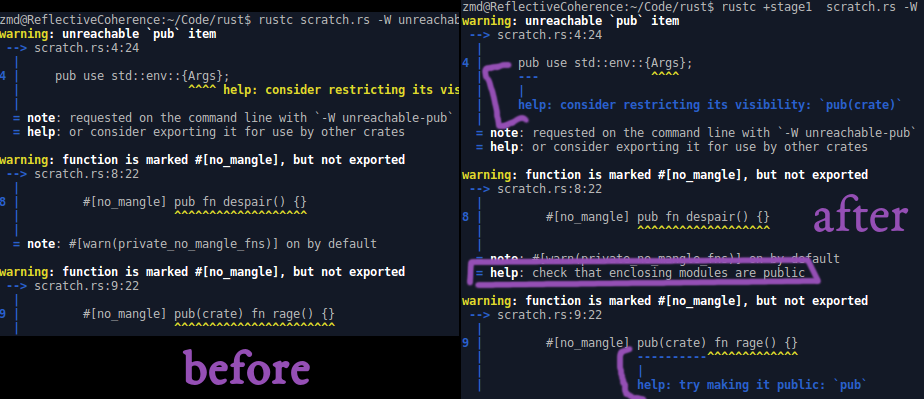
r? @nrc
cc @Manishearth
Loosened rules involving statics mentioning other statics
Before this PR, trying to mention a static in any way other than taking a reference to it caused a compile-time error. So, while
```rust
static A: u32 = 42;
static B: &u32 = &A;
```
compiles successfully,
```rust
static A: u32 = 42;
static B: u32 = A; // error
```
and
```rust
static A: u32 = 42;
static B: u32 = *&A; // error
```
are not possible to express in Rust. On the other hand, introducing an intermediate `const fn` can presently allow one to do just that:
```rust
static A: u32 = 42;
static B: u32 = foo(&A); // success!
const fn foo(a: &u32) -> u32 {
*a
}
```
Preventing `const fn` from allowing to work around the ban on reading from statics would cripple `const fn` almost into uselessness.
Additionally, the limitation for reading from statics comes from the old const evaluator(s) and is not shared by `miri`.
This PR loosens the rules around use of statics to allow statics to evaluate other statics by value, allowing all of the above examples to compile and run successfully.
Reads from extern (foreign) statics are however still disallowed by miri, because there is no compile-time value to be read.
```rust
extern static A: u32;
static B: u32 = A; // error
```
This opens up a new avenue of potential issues, as a static can now not just refer to other statics or read from other statics, but even contain references that point into itself.
While it might seem like this could cause subtle bugs like allowing a static to be initialized by its own value, this is inherently impossible in miri.
Reading from a static causes the `const_eval` query for that static to be invoked. Calling the `const_eval` query for a static while already inside the `const_eval` query of said static will cause cycle errors.
It is not possible to accidentally create a bug in miri that would enable initializing a static with itself, because the memory of the static *does not exist* while being initialized.
The memory is not uninitialized, it is not there. Thus any change that would accidentally allow reading from a not yet initialized static would cause ICEs.
Tests have been modified according to the new rules, and new tests have been added for writing to `static mut`s within definitions of statics (which needs to fail), and incremental compilation with complex/interlinking static definitions.
Note that incremental compilation did not need to be adjusted, because all of this was already possible before with workarounds (like intermediate `const fn`s) and the encoding/decoding already supports all the possible cases.
r? @eddyb
Fix some doc links
The futures crate CI always fails because of these intra doc links. I hope that this will fix this issue.
r? @steveklabnik
@cramertj
Edit: I added @steveklabnik as reviewer because this PR also adjusts a link in `src/libstd/error.rs`
Stabilize Iterator::flatten in 1.29, fixes#48213.
This PR stabilizes [`Iterator::flatten`](https://doc.rust-lang.org/nightly/std/iter/trait.Iterator.html#method.flatten) in *version 1.29* (1.28 goes to beta in 10 days, I don't think there's enough time to land it in that time, but let's see...).
Tracking issue is: #48213.
cc @bluss re. itertools.
r? @SimonSapin
ping @pietroalbini -- let's do a crater run when this passes CI :)
Speed up compilation of large constant arrays
This is a different approach to #51672 as suggested by @oli-obk. Rather
than write each repeated value one-by-one, we write the first one and
then copy its value directly into the remaining memory.
With this change, the [toy program](c2f4744d2d/src/test/run-pass/mir_heavy_promoted.rs) goes from 63 seconds to 19 seconds on my machine.
Edit: Inlining `Size::bytes()` saves an additional 6 seconds dropping the total time to 13 seconds on my machine.
Edit2: Now down to 2.8 seconds.
r? @oli-obk
cc @nnethercote @eddyb
It was pointed out in review that the glob-exported
underscore-suffixed convention for `Spanned` HIR nodes is no longer
preferred: see February 2016's #31487 for AST's migration away from
this style towards properly namespaced NodeKind enums.
This concerns #51968.
NLL: bad error message when converting anonymous lifetime to `'static`
Contributes to #46983. This PR doesn't introduce fantastic errors, but it should hopefully lay some groundwork for diagnostic improvements.
r? @nikomatsakis
Suggest correct comparison against negative literal
When parsing as emplacement syntax (`x<-1`), suggest the correct syntax
for comparison against a negative value (`x< -1`).
Fix#45651.
A fix for 51821
This dedupe the vec of `OutlivesConstraint` using a `FxHashSet<(RegionVid, RegionVid)>` it alsow adds a `struct ConstraintSet` to encapsulate/ensure this behavere.