* make stuff more type-safe by using `BindPat` instead of just `Pat`
* don't add `mut` into binding hash
* reset shadow counter when we enter a function
1537: Less magic completions r=matklad a=marcogroppo
Restrict `if`, `not` and `while` postfix magic completions to boolean expressions and expressions of an unknown type.
(this may be controversial, marking as draft for this reason)
See the discussion in #1526.
Co-authored-by: Marco Groppo <marco.groppo@gmail.com>
This wasn't a right decision in the first place, the feature flag was
broken in the last rustfmt release, and syntax highlighting of imports
is more important anyway
1456: Deduplicate method candidates r=matklad a=flodiebold
With trait method completion + autoderef, we were getting a lot of duplicates, which was really annoying...
Co-authored-by: Florian Diebold <flodiebold@gmail.com>
Can be used like this:
```
$ cargo run --release -p ra_cli -- \
analysis-bench ../chalk/ \
--complete ../chalk/chalk-engine/src/logic.rs:94:0
loading: 225.970093ms
from scratch: 8.492373325s
no change: 445.265µs
trivial change: 95.631242ms
```
Or like this:
```
$ cargo run --release -p ra_cli -- \
analysis-bench ../chalk/ \
--highlight ../chalk/chalk-engine/src/logic.rs
loading: 209.873484ms
from scratch: 9.504916942s
no change: 7.731119ms
trivial change: 124.984039ms
```
"from scratch" includes initial analysis of the relevant bits of the
project
"no change" just asks the same question for the second time. It
measures overhead on assembling the answer outside of salsa.
"trivial change" doesn't do an actual salsa change, it just advances
the revision. This test how fast is salsa at validating things.
1408: Associated type basics & Deref support r=matklad a=flodiebold
This adds the necessary Chalk integration to handle associated types and uses it to implement support for `Deref` in the `*` operator and autoderef; so e.g. dot completions through an `Arc` work now.
It doesn't yet implement resolution of associated types in paths, though. Also, there's a big FIXME about handling variables in the solution we get from Chalk correctly.
Co-authored-by: Florian Diebold <flodiebold@gmail.com>
Note that we can't just remove CheckCanceled trait altogether:
sometimes it's useful to check for cancellation while the query is
running! We do this, for example, in the name resolution fixed-point
loop.
1394: Fix hover for pat that shadows items r=matklad a=sinkuu
```rust
fn x() {}
fn y() {
let x = 0i32;
x; // hover on `x` is expected to be `i32`, but the actual result was `fn x()`
}
```
This was because: if [`res.is_empty()`](656a0fa9f9/crates/ra_ide_api/src/hover.rs (L205)), it fallbacks to "index based approach" and adds `fn x()` to `res`, which makes [`res.extend(type_of)` below](656a0fa9f9/crates/ra_ide_api/src/hover.rs (L260-L266)) not happen.
Co-authored-by: Shotaro Yamada <sinkuu@sinkuu.xyz>
Before this commit, `Parse`s for original file ended up two times in
salsa's db: first, when we parse original file, and second, when we
parse macro or a file.
Given that parse trees are the worst ofenders in terms of memory, it
makes sense to make sure we store them only once.
This small fix should improve rust-analyzer resopnsivness for
real-time operations like onEnter handling.
Turns out, salsa's validation can take hundreds of milliseconds, and,
in case no changes were made, it won't be triggering any queries.
Because we check for cancellation in queries, that means that
validation is not cancellable!
What this PR does is injecting check_canceled checks into validation,
by using salsa's event API, which wasn't meant to be used like this,
but, hey, it works!
Here's the onEnter handling before and after this change:
https://youtu.be/7-ffPzgvH7o
1337: Move syntax errors our of syntax tree r=matklad a=matklad
I am not really sure if it's a good idea, but `SyntaxError` do not really belong to a `SyntaxTree`. So let's just store them on the side?
Co-authored-by: Aleksey Kladov <aleksey.kladov@gmail.com>
Very simple approach: For each identifier, set the hash of the range
where it's defined as its 'id' and use it in the VSCode extension to
generate unique colors.
Thus, the generated colors are per-file. They are also quite fragile,
and I'm not entirely sure why. Looks like we need to make sure the
same ranges aren't overwritten by a later request?
1299: Use ThemeColor and add support for light themes r=matklad a=lnicola
Part of #1294.
- switch to `ThemeColor`
- add light and high contrast theme definitions
- highlight control flow keywords and `unsafe`
Co-authored-by: Laurențiu Nicola <lnicola@dend.ro>
1208: [WIP] Goto for Macro's r=matklad a=Lapz
Adds goto definition for macros. Currently only works for macros in the current crate ~~otherwise it panics~~. Proper macro resolution needs to be added for it to resolve macros in other crates.
Todo
- [X] Allow goto from macro calls
- [X] Fix panics
- [x] Add tests
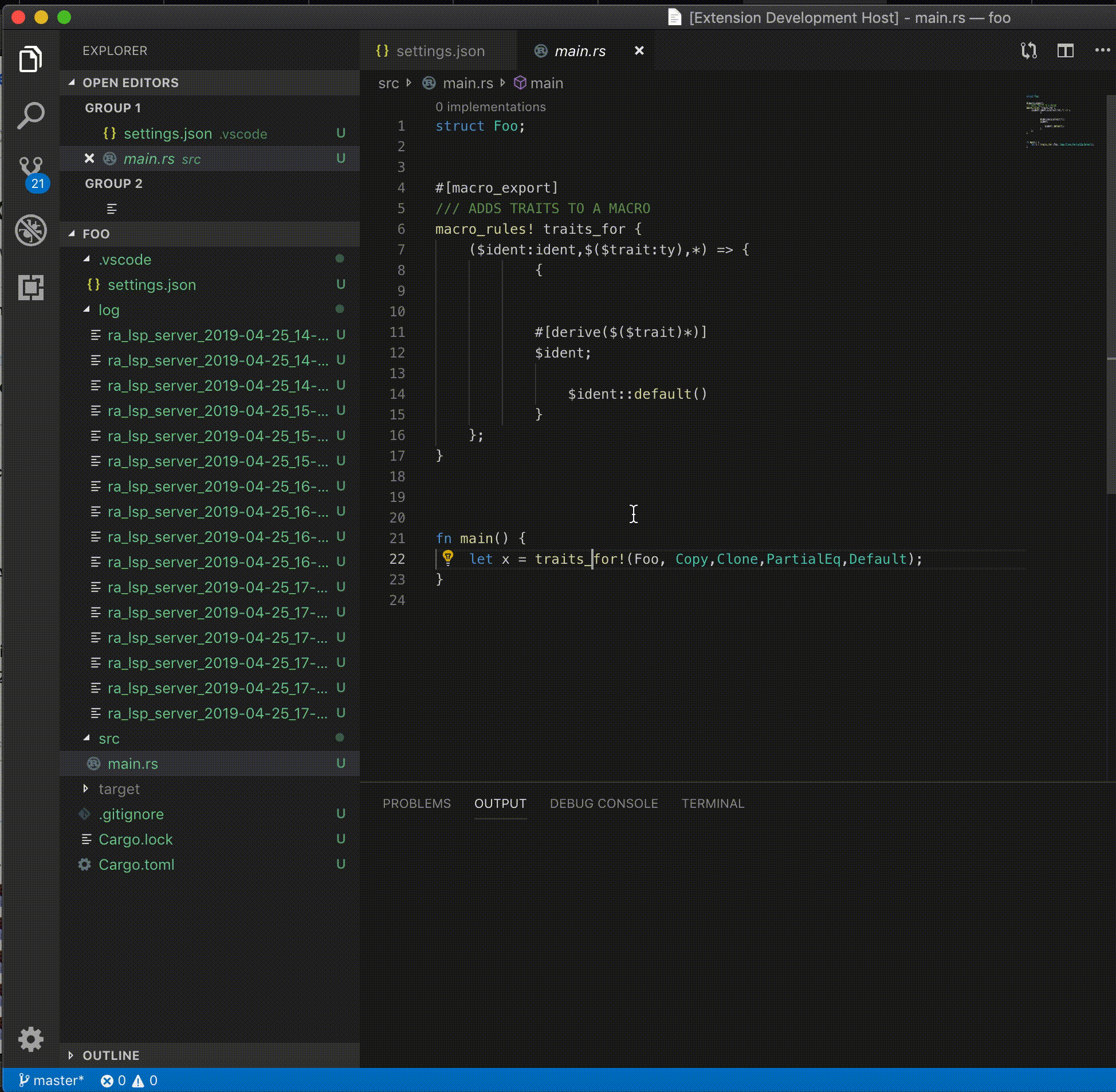
Co-authored-by: Lenard Pratt <l3np27@gmail.com>
E.g. in
```
let foo = 1u32;
if true {
<|>foo;
}
```
the hover shows `()`, the type of the whole if expression, instead of the more
sensible `u32`. The reason for this was that the search for an expression was
slightly left-biased: When on the edge between two tokens, it first looked at
all ancestors of the left token and then of the right token. Instead merge the
ancestors in ascending order, so that we get the smaller of the two possible
expressions.
1200: Allows searching for case-equivalent symbols (fixes#1151) r=matklad a=jrvidal
I couldn't find a nice, functional way of calculating the ranges in one pass so I resorted to a plain old `for` loop.
Co-authored-by: Roberto Vidal <vidal.roberto.j@gmail.com>
We really shouldn't be looking at the identifier at point. Instead,
all filtering and sorting should be implemented at the layer above.
This layer should probably be home for auto-import completions as
well, but, since that is not yet implemented, let's just stick this
into complete_scope.
This fixes the order in which candidates are chosen a bit (not completely
though, as the ignored test demonstrates), and makes autoderef work with trait
methods. As a side effect, this also makes completion of trait methods work :)
1067: Take number of arguments at the call-site into account for signature help r=matklad a=kjeremy
Fixes#1065
Co-authored-by: kjeremy <kjeremy@gmail.com>
1034: HIR diagnostics API r=matklad a=matklad
This PR introduces diagnostics API for HIR, so we can now start issuing errors and warnings! Here are requirements that this solution aims to fulfill:
* structured diagnostics: rather than immediately rendering error to string, we provide a well-typed blob of data with error-description. These data is used by IDE to provide fixes
* open set diagnostics: there's no single enum with all possible diagnostics, which hopefully should result in better modularity
The `Diagnostic` trait describes "a diagnostic", which can be downcast to a specific diagnostic kind. Diagnostics are expressed in terms of macro-expanded syntax tree: they store pointers to syntax nodes. Diagnostics are self-contained: you don't need any context, besides `db`, to fully understand the meaning of a diagnostic.
Because diagnostics are tied to the source, we can't store them in salsa. So subsystems like type-checking produce subsystem-local diagnostic (which is a closed `enum`), which is expressed in therms of subsystem IR. A separate step converts these proto-diagnostics into `Diagnostic`, by merging them with source-maps.
Note that this PR stresses type-system quite a bit: we now type-check every function in open files to compute errors!
Discussion on Zulip: https://rust-lang.zulipchat.com/#narrow/stream/185405-t-compiler.2Fwg-rls-2.2E0/topic/Diagnostics.20API
Co-authored-by: Aleksey Kladov <aleksey.kladov@gmail.com>