internal: add `as_slice` to `hir::Type`
~`remove_slice`~ `as_slice` is same as `remove_ref` but for slices.
Though there is `as_array` which I believe was named such because it also gets the length of the array, maybe. I am still shaky on the names feel free to suggest corrections.
internal: Rename `hir::diagnostics::MissingMatchArms.match_expr` field
`hir::diagnostics::MissingMatchArms.match_expr` had confusing name: it is pointing to scrutinee expression. Renamed to `scrutinee_expr` and used better fitting type for it.
Also small refactorings/cleanup.
MIR episode 2
This PR adds:
1. `need-mut` and `unused-mut` diagnostics
2. `View mir` command which shows MIR for the body under cursor, useful for debugging
3. MIR lowering for or-patterns and for-loops
Handle trait alias definitions
Part of #2773
This PR adds a bunch of structs and enum variants for trait aliases. Trait aliases should be handled as an independent item because they are semantically distinct from traits.
I basically started by adding `TraitAlias{Id, Loc}` to `hir_def::item_tree` and iterated adding necessary stuffs until compiler stopped complaining what's missing. Let me know if there's still anything I need to add.
I'm opening up this PR for early review and stuff. I'm planning to add tests for IDE functionalities in this PR, but not type-related support, for which I put FIXME notes.
Fix associated item visibility in block-local impls
Fixes#14046
When we're resolving visibility of block-local items...
> `self` normally refers to the containing non-block module, and `super` to its parent (etc.). However, visibilities must only refer to a module in the DefMap they're written in, so we restrict them when that happens. ([link])
...unless we're resolving visibility of associated items in block-local impls, because that impl is semantically "hoisted" to the nearest (non-block) module. With this PR, we skip the adjustment for such items.
Since visibility representation of those items is modified, this PR also adjusts visibility rendering in `HirDisplay`.
[link]: a6603fc21d/crates/hir-def/src/nameres/path_resolution.rs (L101-L103)
Fix: Run doctests for structs with lifetime parameters from IDE
Fixes#14142: Doctests can't be triggered for structs with lifetimes
This MR adds lifetime parameters to the structs path for runnables so that they can be triggered from an IDE as well.
This is my first MR for rust-analyzer, please let me know if I should change something, either in code or the description here.
Beginning of MIR
This pull request introduces the initial implementation of MIR lowering and interpreting in Rust Analyzer.
The implementation of MIR has potential to bring several benefits:
- Executing a unit test without compiling it: This is my main goal. It can be useful for quickly testing code changes and print-debugging unit tests without the need for a full compilation (ideally in almost zero time, similar to languages like python and js). There is a probability that it goes nowhere, it might become slower than rustc, or it might need some unreasonable amount of memory, or we may fail to support a common pattern/function that make it unusable for most of the codes.
- Constant evaluation: MIR allows for easier and more correct constant evaluation, on par with rustc. If r-a wants to fully support the type system, it needs full const eval, which means arbitrary code execution, which needs MIR or something similar.
- Supporting more diagnostics: MIR can be used to detect errors, most famously borrow checker and lifetime errors, but also mutability errors and uninitialized variables, which can be difficult/impossible to detect in HIR.
- Lowering closures: With MIR we can find out closure capture modes, which is useful in detecting if a closure implements the `FnMut` or `Fn` traits, and calculating its size and data layout.
But the current PR implements no diagnostics and doesn't support closures. About const eval, I removed the old const eval code and it now uses the mir interpreter. Everything that is supported in stable rustc is either implemented or is super easy to implement. About interpreting unit tests, I added an experimental config, disabled by default, that shows a `pass` or `fail` on hover of unit tests (ideally it should be a button similar to `Run test` button, but I didn't figured out how to add them). Currently, no real world test works, due to missing features including closures, heap allocation, `dyn Trait` and ... so at this point it is only useful for me selecting what to implement next.
The implementation of MIR is based on the design of rustc, the data structures are almost copy paste (so it should be easy to migrate it to a possible future stable-mir), but the lowering and interpreting code is from me.
add: clean api to get `raw_ptr` type
There doesn't seem to be an API to fetch the type of `raw_ptr`, which is helpful for a project I work on.
Notes:
- I am unsure about the function name, do let me know if I should use something else.
- Also unsure about where to add tests, for hir changes. Will fix it as needed.
fix:add a case in which remainig is None in resolveing types when resolving hir path.
fix#14030 The variable type is being determined incorrectly
This PR fixed a problem in which `go to definition` is jumping to the incorrect position because it was failing to resolve the type in case it defined in the module when resolving hir.
In addition, I added a test for this issue and refactored the related code.
This is my first PR and I am using a translation tool to write this text. Let me know if you have any problems.
Support generic function in `generate_function` assist
Part of #3639
This PR adds support for generic function generation in `generate_function` assist. Now the assist looks for generic parameters and trait bounds in scope, filters out irrelevant ones, and generates new function with them.
See `fn_generic_params()` for the outline of the procedure, and see comments on `filter_unnecessary_bounds()` for criteria for filtering. I think it's good criteria for most cases, but I'm open to opinions and suggestions.
The diff is pretty big, but it should run in linear time w.r.t. the number of nodes we operate on and should be fast enough.
Some notes:
- When we generate function in an existing impl, generic parameters may cause name conflict. While we can detect the conflict and rename conflicting params, I didn't find it worthwhile mainly because it's really easy to resolve on IDE: use Rename functionality.
- I've implemented graph structure myself, because we don't have graph library as a dependency and we only need the simplest one.
- Although `petgraph` is in our dependency graph and I was initially looking to use it, we don't actually depend on it AFAICT since it's only used in chalk's specialization graph handling, which we don't use. I'd be happy to replace my implementation with `petgraph` if it's okay to use it though.
- There are some caveats that I consider out of scope of this PR. See FIXME notes on added tests.
add wrapping/checked/saturating assist
This addresses #13452
I'm not sure about the structure of the code. I'm not sure if it needs to be 3 separate assists, and if that means it needs to be in 3 separate files as well.
Most of the logic is in `util.rs`, which feels funny to me, but there seems to be a pattern of 1 assist per file, and this seems better than duplicating the logic.
Let me know if anything needs changes 😁
This makes code more readale and concise,
moving all format arguments like `format!("{}", foo)`
into the more compact `format!("{foo}")` form.
The change was automatically created with, so there are far less change
of an accidental typo.
```
cargo clippy --fix -- -A clippy::all -W clippy::uninlined_format_args
```
Seems like these can be safely fixed. With one, I was particularly
surprised -- `Some(pats) => &**pats,` in body.rs?
```
cargo clippy --fix -- -A clippy::all -D clippy::explicit_auto_deref
```
I am not certain if this will improve performance,
but it seems having a .clone() without any need should be removed.
This was done with clippy, and manually reviewed:
```
cargo clippy --fix -- -A clippy::all -D clippy::redundant_clone
```
feat: Add an option to hide adjustment hints outside of `unsafe` blocks and functions
As the title suggests: this PR adds an option (namely `rust-analyzer.inlayHints.expressionAdjustmentHints.hideOutsideUnsafe`) that allows to hide adjustment hints outside of `unsafe` blocks and functions:
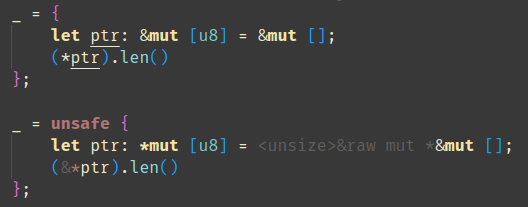
Requested by `@BoxyUwU` <3
fix: only shift `BoundVar`s that come from outside lowering context
Fixes#13734
There are some free functions `TyLoweringContext` methods call, which do not know anything about current binders in scope. We need to shift in the `BoundVar`s in substitutions that we get from them (#4952), but not those we get from `TyLoweringContext` methods.
Compute data layout of types
cc #4091
Things that aren't working:
* Closures
* Generators (so no support for `Future` I think)
* Opaque types
* Type alias and associated types which may need normalization
Things that show wrong result:
* ~Enums with explicit discriminant~
* SIMD types
* ~`NonZero*` and similar standard library items which control layout with special attributes~
At the user level, I didn't put much work, since I wasn't confident about what is the best way to present this information. Currently it shows size and align for ADTs, and size, align, offset for struct fields, in the hover, similar to clangd. I used it some days and I feel I liked it, but we may consider it too noisy and move it to an assist or command.
feat: Display the value of enum variant on hover
fixes#12955
This PR adds const eval support for enums, as well as showing their value on hover, just as consts currently have.
I developed these two things at the same time, but I've realized now that they are separate. However since the hover is just a 10 line change (not including tests), I figured I may as well put them in the same PR. Though if you want them split up into "enum const eval support" and "show enum variant value on hover", I think that's reasonable too.
Since this adds const eval support for enums this also allows consts that reference enums to have their values computed now too.
The const evaluation itself is quite rudimentary, it doesn't keep track of the actual type of the enum, but it turns out that Rust doesn't actually either, and `E::A as u8` is valid regardless of the `repr` on `E`.
It also doesn't really care about what expression the enum variant contains, it could for example be a string, despite that not being allowed, but I guess it's up to the `cargo check` diagnostics to inform of such issues anyway?
fixup: remove unnecessary `Option`
Fixup for #13223, two things:
- `normalize_projection_query()` (and consequently `HirDatabase::normalize_projection()`) never returns `None` (well, it used to when I first wrote it...), so just return `Ty` instead of `Option<Ty>`
- When chalk cannot normalize projection uniquely, `normalize_trait_assoc_type()` used to return `None` before #13223, but not anymore because of the first point. I restored the behavior so its callers work as before.
fix: handle lifetime variables in projection normalization
Fixes#12674
The problem is that we've been skipping the binders of normalized projections assuming they should be empty, but the assumption is unfortunately wrong. We may get back lifetime variables and should handle them before returning them as normalized projections. For those who are curious why we get those even though we treat all lifetimes as 'static, [this comment in chalk](d875af0ff1/chalk-solve/src/infer/unify.rs (L888-L908)) may be interesting.
I thought using `InferenceTable` would be cleaner than the other ways as it already has the methods for canonicalization, normalizing projection, and resolving variables, so moved goal building and trait solving logic to a new `HirDatabase` query. I made it transparent query as the query itself doesn't do much work but the eventual call to `HirDatabase::trait_solve_query()` does.
This hir expression isn't needed and only existed as it was simpler to
deal with at first as it gave us a direct mapping for the ast version of
the same construct. This PR removes it, properly handling the statements
that are introduced by macro call expressions.
internal: Record all macro definitions in ItemScope
Fixes https://github.com/rust-lang/rust-analyzer/issues/12100
Doesn't resolve the shadowing issues though, fixing those is gonna be really tricky I believe unless we can come up with a nice scheme to "order" item tree items (using syntax ranges and file ids would be a pain and also a bad idea since that'll require us to potentially reparse files in collection).
This change attempts to resolve issue #7636: Extract into Function does not
create a generic function with constraints when extracting generic code.
In `FunctionBody::analyze_container`, we now traverse the `ancestors` in search
of `AnyHasGenericParams`, and attach any `GenericParamList`s and `WhereClause`s
we find to the `ContainerInfo`.
Later, in `format_function`, we collect all the `GenericParam`s and
`WherePred`s from the container, and filter them to keep only types matching
`TypeParam`s used within the newly extracted function body or param list. We
can then include the new `GenericParamList` and `WhereClause` in the new
function definition.
This change only impacts `TypeParam`s. `LifetimeParam`s and `ConstParam`s are
out of scope for this change.
feat: Show witnesses of non-exhaustiveness in `missing-match-arm` diagnostic
Shamelessly copied from rustc. Thus reporting format is same.
This extends public api `hir::diagnostics::MissingMatchArms` with `uncovered_patterns: String` field. It does not expose data for implementing a quick fix yet.
-----
Worth to note: current implementation does not give a comprehensive list of missing patterns. Also mentioned in [paper](http://moscova.inria.fr/~maranget/papers/warn/warn.pdf):
> One may think that algorithm I should make an additional effort to provide more
> non-matching values, by systematically computing recursive calls on specialized
> matrices when possible, and by returning a list of all pattern vectors returned by
> recursive calls. We can first observe that it is not possible in general to supply the
> users with all non-matching values, since the signature of integers is (potentially)
> infinite.
Distinguish between
- there is no build data (for some reason?)
- there is build data, but the cargo package didn't build a proc macro dylib
- there is a proc macro dylib, but it didn't contain the proc macro we expected
- the name did not resolve to any macro (this is now an
unresolved_macro_call even for attributes)
I changed the handling of disabled attribute macro expansion to
immediately ignore the macro and report an unresolved_proc_macro,
because otherwise they would now result in loud unresolved_macro_call
errors. I hope this doesn't break anything.
Also try to improve error ranges for unresolved_macro_call / macro_error
by reusing the code for unresolved_proc_macro. It's not perfect but
probably better than before.
- remove Valid, it serves no purpose and just obscures the diff
- rename some things
- don't use is_valid_candidate when searching for impl, it's not necessary
fix: doc url link type
fix: #12033
I did some debugging and found the cause looks like to be some doc links' `LinkType` are kept as `Shortcut` which don't make sense for url links.
This PR should resolve both problems in the origin issue, but aside this PR, more work are needed for doc_links.
about `LinkType`: f29bd1e228/src/lib.rs (L191-L210)
11925: internal: Add and use `HirFormatter::write_{str,char}` r=Veykril a=lnicola
Saves slightly over 3 KB of `text`, but comparing the total with that from two weeks ago in #11776, this is a losing battle (we're 951 KB larger).
```
text data bss dec hex filename
24693512 1542704 4424 26240640 1906680 rust-analyzer-baseline
24690216 1542112 4424 26236752 1905750 rust-analyzer-pr
```
Co-authored-by: Laurențiu Nicola <lnicola@dend.ro>