Optimize Vec::retain
Use `copy_non_overlapping` instead of `swap` to reduce memory writes, like what we've done in #44355 and `String::retain`.
#48065 already tried to do this optimization but it is reverted in #67300 due to bad codegen of `DrainFilter::drop`.
This PR re-implement the drop-then-move approach. I did a [benchmark](https://gist.github.com/oxalica/3360eec9376f22533fcecff02798b698) on small-no-drop, small-need-drop, large-no-drop elements with different predicate functions. It turns out that the new implementation is >20% faster in average for almost all cases. Only 2/24 cases are slower by 3% and 5%. See the link above for more detail.
I think regression in may-panic cases is due to drop-guard preventing some optimization. If it's permitted to leak elements when predicate function of element's `drop` panic, the new implementation should be almost always faster than current one.
I'm not sure if we should leak on panic, since there is indeed an issue (#52267) complains about it before.
Bump stabilization version for const int methods
These methods missed the beta cutoff. See #80962 for details.
`@rustbot` modify labels to +A-const-fn, +A-intrinsics
r? `@m-ou-se`
Make Vec::split_at_spare_mut public
This PR introduces a new method to the public API, under
`vec_split_at_spare` feature gate:
```rust
impl<T, A: Allocator> impl Vec<T, A> {
pub fn split_at_spare_mut(&mut self) -> (&mut [T], &mut [MaybeUninit<T>]);
}
```
The method returns 2 slices, one slice references the content of the vector,
and the other references the remaining spare capacity.
The method was previously implemented while adding `Vec::extend_from_within` in #79015,
and used to implement `Vec::spare_capacity_mut` (as the later is just a
subset of former one).
See also previous [discussion in `Vec::spare_capacity_mut` tracking issue](https://github.com/rust-lang/rust/issues/75017#issuecomment-770381335).
## Unresolved questions
- [ ] Should we consider changing the name? `split_at_spare_mut` doesn't seem like an intuitive name
- [ ] Should we deprecate `Vec::spare_capacity_mut`? Any usecase of `Vec::spare_capacity_mut` can be replaced with `Vec::split_at_spare_mut` (but not vise-versa)
r? `@KodrAus`
Add `Box::into_inner`.
This adds a `Box::into_inner` method to the `Box` type. <del>I actually suggest deprecating the compiler magic of `*b` if this gets stablized in the future.</del>
r? `@m-ou-se`
Rollup of 11 pull requests
Successful merges:
- #72209 (Add checking for no_mangle to unsafe_code lint)
- #80732 (Allow Trait inheritance with cycles on associated types take 2)
- #81697 (Add "every" as a doc alias for "all".)
- #81826 (Prefer match over combinators to make some Box methods inlineable)
- #81834 (Resolve typedef in HashMap lldb pretty-printer only if possible)
- #81841 ([rustbuild] Output rustdoc-json-types docs )
- #81849 (Expand the docs for ops::ControlFlow a bit)
- #81876 (parser: Fix panic in 'const impl' recovery)
- #81882 (⬆️ rust-analyzer)
- #81888 (Fix pretty printer macro_rules with semicolon.)
- #81896 (Remove outdated comment in windows' mutex.rs)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
Remove outdated comment in windows' mutex.rs
After https://github.com/rust-lang/rust/pull/81250, this `Mutex` no longer falls back to the `ReentrantMutex` implementation, so this comment is no longer relevant.
Expand the docs for ops::ControlFlow a bit
Since I was writing some examples for an RFC anyway.
And I almost made the mistake of reordering the variants, so added a note and a test about that.
Prefer match over combinators to make some Box methods inlineable
Hopefully this patch would make two snippets generated identical code: <https://rust.godbolt.org/z/fjrj4E>.
libtest: allow multiple filters
Libtest ignores any filters after the first. This changes it so that if multiple filters are passed, it will test against all of them.
This also affects compiletest to do the same.
Closes#30422
BTree: remove Ord bound where it is absent elsewhere
Some btree methods don't really need an Ord bound and don't have one, while some methods that more obviously don't need it, do have one.
An example of the former is `iter`, even though it explicitly exposes the work of the Ord implementation (["sorted by key"](https://doc.rust-lang.org/std/collections/struct.BTreeMap.html#method.iter) - but I'm not suggesting it should have the Ord bound). An example of the latter is `new`, which doesn't involve any keys whatsoever.
Stabilize remaining integer methods as `const fn`
This pull request stabilizes the following methods as `const fn`:
- `i*::checked_div`
- `i*::checked_div_euclid`
- `i*::checked_rem`
- `i*::checked_rem_euclid`
- `i*::div_euclid`
- `i*::overflowing_div`
- `i*::overflowing_div_euclid`
- `i*::overflowing_rem`
- `i*::overflowing_rem_euclid`
- `i*::rem_euclid`
- `i*::wrapping_div`
- `i*::wrapping_div_euclid`
- `i*::wrapping_rem`
- `i*::wrapping_rem_euclid`
- `u*::checked_div`
- `u*::checked_div_euclid`
- `u*::checked_rem`
- `u*::checked_rem_euclid`
- `u*::div_euclid`
- `u*::overflowing_div`
- `u*::overflowing_div_euclid`
- `u*::overflowing_rem`
- `u*::overflowing_rem_euclid`
- `u*::rem_euclid`
- `u*::wrapping_div`
- `u*::wrapping_div_euclid`
- `u*::wrapping_rem`
- `u*::wrapping_rem_euclid`
These can all be implemented on the current stable (1.49). There are two unstable details: const likely/unlikely and unchecked division/remainder. Both of these are for optimizations, and are in no way required to make the methods function; there is no exposure of these details publicly. Per comments below, it seems best practice is to stabilize the intrinsics. As such, `intrinsics::unchecked_div` and `intrinsics::unchecked_rem` have been stabilized as `const` as part of this pull request as well. The methods themselves remain unstable.
I believe part of the reason these were not stabilized previously was the behavior around division by 0 and modulo 0. After testing on nightly, the diagnostic for something like `const _: i8 = 5i8 % 0i8;` is similar to that of `const _: i8 = 5i8.rem_euclid(0i8);` (assuming the appropriate feature flag is enabled). As such, I believe these methods are ready to be stabilized as `const fn`.
This pull request represents the final methods mentioned in #53718. As such, this PR closes#53718.
`@rustbot` modify labels to +A-const-fn, +T-libs
expand/resolve: Turn `#[derive]` into a regular macro attribute
This PR turns `#[derive]` into a regular attribute macro declared in libcore and defined in `rustc_builtin_macros`, like it was previously done with other "active" attributes in https://github.com/rust-lang/rust/pull/62086, https://github.com/rust-lang/rust/pull/62735 and other PRs.
This PR is also a continuation of #65252, #69870 and other PRs linked from them, which layed the ground for converting `#[derive]` specifically.
`#[derive]` still asks `rustc_resolve` to resolve paths inside `derive(...)`, and `rustc_expand` gets those resolution results through some backdoor (which I'll try to address later), but otherwise `#[derive]` is treated as any other macro attributes, which simplifies the resolution-expansion infra pretty significantly.
The change has several observable effects on language and library.
Some of the language changes are **feature-gated** by [`feature(macro_attributes_in_derive_output)`](https://github.com/rust-lang/rust/issues/81119).
#### Library
- `derive` is now available through standard library as `{core,std}::prelude::v1::derive`.
#### Language
- `derive` now goes through name resolution, so it can now be renamed - `use derive as my_derive; #[my_derive(Debug)] struct S;`.
- `derive` now goes through name resolution, so this resolution can fail in corner cases. Crater found one such regression, where import `use foo as derive` goes into a cycle with `#[derive(Something)]`.
- **[feature-gated]** `#[derive]` is now expanded as any other attributes in left-to-right order. This allows to remove the restriction on other macro attributes following `#[derive]` (https://github.com/rust-lang/reference/issues/566). The following macro attributes become a part of the derive's input (this is not a change, non-macro attributes following `#[derive]` were treated in the same way previously).
- `#[derive]` is now expanded as any other attributes in left-to-right order. This means two derive attributes `#[derive(Foo)] #[derive(Bar)]` are now expanded separately rather than together. It doesn't generally make difference, except for esoteric cases. For example `#[derive(Foo)]` can now produce an import bringing `Bar` into scope, but previously both `Foo` and `Bar` were required to be resolved before expanding any of them.
- **[feature-gated]** `#[derive()]` (with empty list in parentheses) actually becomes useful. For historical reasons `#[derive]` *fully configures* its input, eagerly evaluating `cfg` everywhere in its target, for example on fields.
Expansion infra doesn't do that for other attributes, but now when macro attributes attributes are allowed to be written after `#[derive]`, it means that derive can *fully configure* items for them.
```rust
#[derive()]
#[my_attr]
struct S {
#[cfg(FALSE)] // this field in removed by `#[derive()]` and not observed by `#[my_attr]`
field: u8
}
```
- `#[derive]` on some non-item targets is now prohibited. This was accidentally allowed as noop in the past, but was warned about since early 2018 (#50092), despite that crater found a few such cases in unmaintained crates.
- Derive helper attributes used before their introduction are now reported with a deprecation lint. This change is long overdue (since macro modularization, https://github.com/rust-lang/rust/issues/52226#issuecomment-422605033), but it was hard to do without fixing expansion order for derives. The deprecation is tracked by #79202.
```rust
#[trait_helper] // warning: derive helper attribute is used before it is introduced
#[derive(Trait)]
struct S {}
```
Crater analysis: https://github.com/rust-lang/rust/pull/79078#issuecomment-731436821
Add a note about the correctness and the effect on unsafe code to the `ExactSizeIterator` docs
As it is a safe trait it does not provide any guarantee that the
returned length is correct and as such unsafe code must not rely on it.
That's why `TrustedLen` exists.
Fixes https://github.com/rust-lang/rust/issues/81739
Upgrade wasm32 image to Ubuntu 20.04
This switches the wasm32 image, which is used to test
wasm32-unknown-emscripten, to Ubuntu 20.04. While at it, enable
most of the excluded tests, as they seem to work fine with some
minor fixes.
This switches the wasm32 image, which is used to test
wasm32-unknown-emscripten to Ubuntu 20.04. While at it, enable
most of the excluded tests, as they seem to work fine with some
minor fixes.
BTreeMap: make Ord bound explicit, compile-test its absence
Most `BTreeMap` and `BTreeSet` members are subject to an `Ord` bound but a fair number of methods are not. To better convey and perhaps later tune the `Ord` bound, make it stand out in individual `where` clauses, instead of once far away at the beginning of an `impl` block. This PR does not introduce or remove any bounds.
Also adds compilation test cases checking that the bound doesn't creep in unintended on the historically unbounded methods.
Revert 78373 ("dont leak return value after panic in drop")
Short term resolution for issue #80949.
Reopen#47949 after this lands.
(We plan to fine-tune PR #78373 to not run into this problem.)
Update LayoutError/LayoutErr stability attributes
`LayoutError` ended up not making it into 1.49.0, updating the stability attributes to reflect that.
I also pushed `LayoutErr` deprecation back a release to allow 2 releases before the deprecation comes into effect.
This change should be backported to beta.
Expose correct symlink API on WASI
As described in https://github.com/rust-lang/rust/issues/68574, the currently exposed API for symlinks is, in fact, a thin wrapper around the corresponding syscall, and not suitable for public usage.
The reason is that the 2nd param in the call is expected to be a handle of a "preopened directory" (a WASI concept for exposing dirs), and the only way to retrieve such handle right now is by tinkering with a private `__wasilibc_find_relpath` API, which is an implementation detail and definitely not something we want users to call directly.
Making matters worse, the semantics of this param aren't obvious from its name (`fd`), and easy to misinterpret, resulting in people trying to pass a handle of the target file itself (as in https://github.com/vitiral/path_abs/pull/50), which doesn't work as expected.
I did a [codesearch among open-source repos](https://sourcegraph.com/search?q=std%3A%3Aos%3A%3Awasi%3A%3Afs%3A%3Asymlink&patternType=literal), and the usage above is so far the only usage of this API at all, but we should fix it before more people start using it incorrectly.
While this is technically a breaking API change, I believe it's a justified one, as 1) it's OS-specific and 2) there was strictly no way to correctly use the previous form of the API, and if someone does use it, they're likely doing it wrong like in the example above.
The new API does not lead to the same confusion, as it mirrors `std::os::unix::fs::symlink` and `std::os::windows::fs::symlink_{file,dir}` variants by accepting source/target paths.
Fixes#68574.
r? ``@alexcrichton``
Stabilize poison API of Once, rename poisoned()
This stabilizes:
* `OnceState`
* `OnceState::is_poisoned()` (previously named `poisoned()`)
* `Once::call_once_force()`
`poisoned()` was renamed because the new name is more clear as a few
people agreed and nobody objected.
Closes#33577
Notes:
* I'm not entirely sure it's supposed to be 1.51, LMK if I did it wrong
* I failed to run tests locally, so we will have to leave it to bors or someone else can try
OsStr eq_ignore_ascii_case takes arg by value
Per a comment on #70516 this changes `eq_ignore_ascii_case` to take the generic parameter `S: AsRef<OsStr>` by value instead of by reference.
This is technically a breaking change to an unstable method. I think the only way it would break is if you called this method with an explicit type parameter, ie `my_os_str.eq_ignore_ascii_case::<str>("foo")` becomes `my_os_str.eq_ignore_ascii_case::<&str>("foo")`.
Besides that, I believe it is overall more flexible since it can now take an owned `OsString` for example.
If this change should be made in some other PR (like #80193) then please just close this.
Add lint for `panic!(123)` which is not accepted in Rust 2021.
This extends the `panic_fmt` lint to warn for all cases where the first argument cannot be interpreted as a format string, as will happen in Rust 2021.
It suggests to add `"{}",` to format the message as a string. In the case of `std::panic!()`, it also suggests the recently stabilized
`std::panic::panic_any()` function as an alternative.
It renames the lint to `non_fmt_panic` to match the lint naming guidelines.
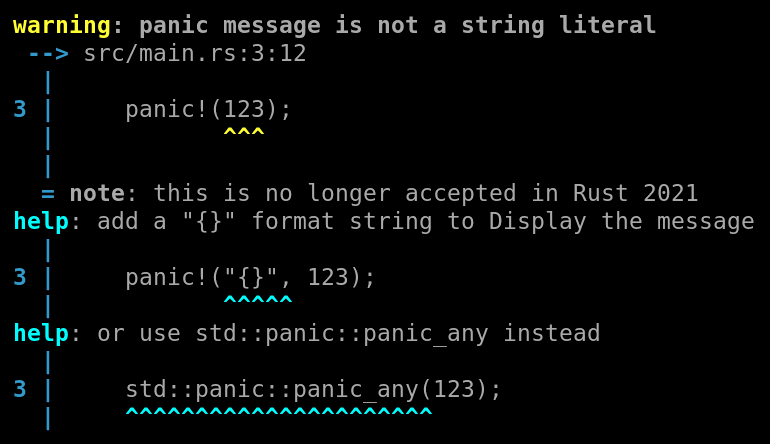
This is part of #80162.
r? ```@estebank```
Rename Iterator::fold_first to reduce and stabilize it
This stabilizes `#![feature(iterator_fold_self)]`.
The name for this function (originally `fold_first`) was still an open question, but the discussion on [the tracking issue](https://github.com/rust-lang/rust/issues/68125) seems to have converged to `reduce`.
This stabilizes:
* `OnceState`
* `OnceState::is_poisoned()` (previously named `poisoned()`)
* `Once::call_once_force()`
`poisoned()` was renamed because the new name is more clear as a few
people agreed and nobody objected.
Closes#33577