unix/weak: pass arguments to syscall at the given type
Given that we know the type the argument should have, it seems a bit strange not to use that information.
r? `@m-ou-se` `@cuviper`
Never inline naked functions
The `#[naked]` attribute disabled prologue / epilogue emission for the
function and it is responsibility of a developer to provide them. The
compiler is no position to inline such functions correctly.
Disable inlining of naked functions at LLVM and MIR level.
Closes#60919.
Add lint for panic!("{}")
This adds a lint that warns about `panic!("{}")`.
`panic!(msg)` invocations with a single argument use their argument as panic payload literally, without using it as a format string. The same holds for `assert!(expr, msg)`.
This lints checks if `msg` is a string literal (after expansion), and warns in case it contained braces. It suggests to insert `"{}", ` to use the message literally, or to add arguments to use it as a format string.
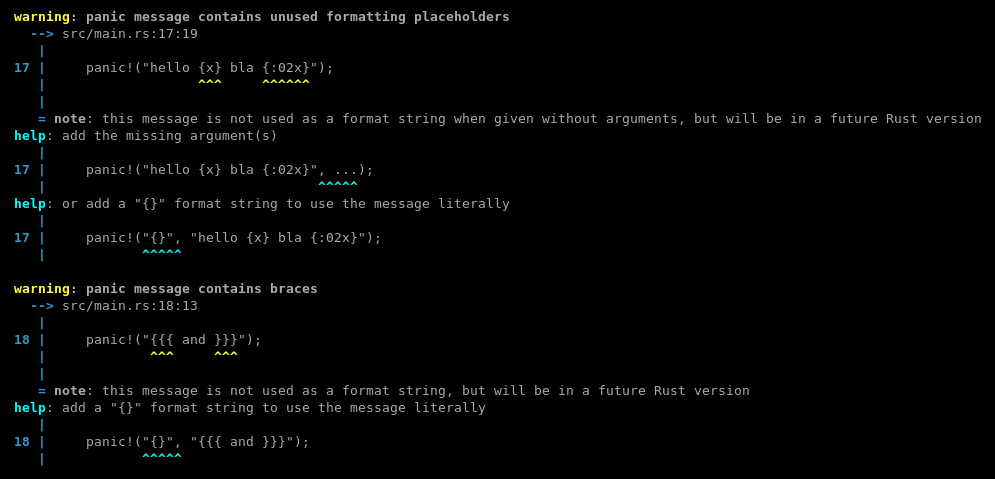
This lint is also a good starting point for adding warnings about `panic!(not_a_string)` later, once [`panic_any()`](https://github.com/rust-lang/rust/pull/74622) becomes a stable alternative.
Rollup of 11 pull requests
Successful merges:
- #79119 (Clarify availability of atomic operations)
- #79123 (Add u128 and i128 integer tests)
- #79177 (Test drop order for (destructuring) assignments)
- #79181 (rustdoc: add [src] links to methods on a trait's page)
- #79183 (Make compiletest testing use the local sysroot)
- #79185 (expand/resolve: Pre-requisites to "Turn `#[derive]` into a regular macro attribute")
- #79193 (Revert #78969 "Normalize function type during validation")
- #79194 (Make as{_mut,}_slice on array::IntoIter public)
- #79204 (Add jyn514 email alias to mailmap)
- #79212 (Move `rustc_ty` -> `rustc_ty_utils`)
- #79217 (Add the "memcpy" doc alias to slice::copy_from_slice)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
The `#[naked]` attribute disabled prologue / epilogue emission for the
function and it is responsibility of a developer to provide them. The
compiler is no position to inline such functions correctly.
Disable inlining of naked functions at LLVM and MIR level.
Make as{_mut,}_slice on array::IntoIter public
The functions are useful in cases where you want to move data out of the IntoIter in bulk, by transmute_copy'ing the slice and then forgetting the IntoIter.
In the compiler, this is useful for providing a sped up IntoIter implementation. One can alternatively provide a separate allocate_array function but one can avoid duplicating some logic by passing everything through the generic iterator using interface.
As per suggestion in https://github.com/rust-lang/rust/pull/78569/files#r526506964
Make compiletest testing use the local sysroot
We already set `compiletest` to use the local sysroot in #68019, but
that missed the configuration for testing `compiletest` itself.
Test drop order for (destructuring) assignments
Add a test that checks whether the drop order of `let` bindings is consistent with the drop order of the corresponding destructuring assignments.
Thanks to ```@RalfJung``` for the suggesting this test ([here](https://github.com/rust-lang/rust/pull/79016#issuecomment-727608732)) and an implementation!
r? ```@RalfJung```
Clarify availability of atomic operations
This was noticed while we were updating the embedded rust book: https://github.com/rust-embedded/book/pull/273/files
These targets do natively have atomic load/stores, but do not support CAS operations.
Rollup of 14 pull requests
Successful merges:
- #78961 (Make bad "rust-call" arguments no longer ICE)
- #79082 (Improve the diagnostic for when an `fn` contains qualifiers inside an `extern` block.)
- #79090 (libary: Forward compiler-builtins "asm" and "mangled-names" feature)
- #79094 (Add //ignore-macos to pretty-std-collections.rs)
- #79101 (Don't special case constant operands when lowering intrinsics)
- #79102 (Add two regression tests)
- #79110 (Remove redundant notes in E0275)
- #79116 (compiletest: Fix a warning in debuginfo tests on windows-gnu)
- #79117 (add optimization fuel checks to some mir passes)
- #79147 (Highlight MIR as Rust on GitHub)
- #79149 (Move capture lowering from THIR to MIR)
- #79155 (fix handling the default config for profiler and sanitizers)
- #79156 (Allow using `download-ci-llvm` from directories other than the root)
- #79164 (Permit standalone generic parameters as const generic arguments in macros)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
fix handling the default config for profiler and sanitizers
#78354 don't handle the case that user don't add any target-specific config in `[target.*]` of `config.toml`:
```toml
changelog-seen = 2
[llvm]
link-shared = true
[build]
sanitizers = true
profiler = true
[install]
[rust]
[dist]
```
The previes code handle the default config in `Config::prase()`:
```rust
target.sanitizers = cfg.sanitizers.unwrap_or(build.sanitizers.unwrap_or_default());
target.profiler = cfg.profiler.unwrap_or(build.profiler.unwrap_or_default());
config.target_config.insert(TargetSelection::from_user(&triple), target);
```
In this case, `toml.target` don't contain any target, so the above code won't execute. Instead, a default `Target` is insert in c919f490bb/src/bootstrap/sanity.rs (L162-L166)
The default value for `bool` is false, hence the issue in #79124
This fix change the type of `sanitizers` and `profiler` to `Option<bool>`, so the default value is `None`, and fallback config is handled in `Config::sanitizers_enabled` and `Config::profiler_enabled`
fix#79124
cc `@Mark-Simulacrum` `@richkadel`
Move capture lowering from THIR to MIR
This allows us to:
- Handle precise Places captured by a closure directly in MIR. Handling
captures in MIR is easier since we can rely on/ tweak PlaceBuilder to
generate `mir::Place`s that resemble how we store captures (`hir::Place`).
- Handle `let _ = x` case when feature `capture_disjoint_fields`
is enabled directly in MIR. This is required to be done in MIR since
patterns are desugared in MIR.
Closes: rust-lang/project-rfc-2229#25
r? ```@nikomatsakis```