Use correct line offsets for doctests
Not yet tested.
This doesn't handle char positions. It could if I collected a map of char offsets and lines, but this is a bit more work and requires hooking into the parser much more (unsure if it's possible).
r? @QuietMisdreavus
(fixes#45868)
Implement libstd for CloudABI.
Though CloudABI is strongly inspired by POSIX, its absence of features that don't work well with capability-based sandboxing makes it different enough that adding bits to `sys/unix` will make things a mess. This change therefore adds CloudABI specific platform code under `sys/cloudabi`.
One of the goals of this implementation is to build as much as possible directly on top of CloudABI's system call layer, as opposed to using the C library. This is preferred, as the system call layer is supposed to be stable, whereas the C library ABI technically is not. An advantage of this approach is that it allows us to implement certain interfaces, such as mutexes and condition variables more optimally. They can be lighter than the ones provided by pthreads.
This change disables some modules that cannot realistically be implemented right now. For example, libstd's pathname abstraction is not designed with POSIX `*at()` (e.g., `openat()`) in mind. The `*at()` functions are the only set of file system APIs available on CloudABI. There is no global file system namespace, nor a process working directory. Discussions on how to port these modules over are outside the scope of this change.
rustc: Tweak `#[target_feature]` syntax
This is an implementation of the `#[target_feature]` syntax-related changes of
[RFC 2045][rfc]. Notably two changes have been implemented:
* The new syntax is `#[target_feature(enable = "..")]` instead of
`#[target_feature = "+.."]`. The `enable` key is necessary instead of the `+`
to indicate that a feature is being enabled, and a sub-list is used for
possible expansion in the future. Additionally within this syntax the feature
names being enabled are now whitelisted against a known set of target feature
names that we know about.
* The `#[target_feature]` attribute can only be applied to unsafe functions. It
was decided in the RFC that invoking an instruction possibly not defined for
the current processor is undefined behavior, so to enable this feature for now
it requires an `unsafe` intervention.
[rfc]: https://github.com/rust-lang/rfcs/blob/master/text/2045-target-feature.md
This is an implementation of the `#[target_feature]` syntax-related changes of
[RFC 2045][rfc]. Notably two changes have been implemented:
* The new syntax is `#[target_feature(enable = "..")]` instead of
`#[target_feature = "+.."]`. The `enable` key is necessary instead of the `+`
to indicate that a feature is being enabled, and a sub-list is used for
possible expansion in the future. Additionally within this syntax the feature
names being enabled are now whitelisted against a known set of target feature
names that we know about.
* The `#[target_feature]` attribute can only be applied to unsafe functions. It
was decided in the RFC that invoking an instruction possibly not defined for
the current processor is undefined behavior, so to enable this feature for now
it requires an `unsafe` intervention.
[rfc]: https://github.com/rust-lang/rfcs/blob/master/text/2045-target-feature.md
No longer parse it.
Remove AutoTrait variant from AST and HIR.
Remove backwards compatibility lint.
Remove coherence checks, they make no sense for the new syntax.
Remove from rustdoc.
Remove deprecated unstable attribute #[simd]
The `#[simd]` attribute has been deprecated since c8b6d5b23c back in 2015. Any nightly crates using it have had ample time to switch to `#[repr(simd)]`, and if they didn't they're likely broken by now anyway.
r? @eddyb
`struct` pattern parsing and diagnostic tweaks
- Recover from struct parse error on match and point out missing match
body.
- Point at struct when finding non-identifier while parsing its fields.
- Add label to "expected identifier, found {}" error.
Fix#15980.
- Simplify nth() by making use of the fact that the slice is evenly
divisible by the chunk size, and calling next() instead of
duplicating it
- Call next_back() in last(), they are equivalent
- Implement ExactSizeIterator::is_empty()
These guarantee that always the requested slice size will be returned
and any leftoever elements at the end will be ignored. It allows llvm to
get rid of bounds checks in the code using the iterator.
This is inspired by the same iterators provided by ndarray.
See https://github.com/rust-lang/rust/issues/47115
Use DefIndex encoding that works better with on-disk variable length integer representations.
Use the least instead of the most significant bit for representing the address space.
r? @eddyb
Fix 45345
There is a fix for https://github.com/rust-lang/rust/issues/45345
It re-introduces `CFG_LIBDIR_RELATIVE` which was broken when migration from `configure` script to `x.py`.
Other commits fix errors which happen after rustbuild cleanups.
type error method suggestions use whitelisted identity-like conversions
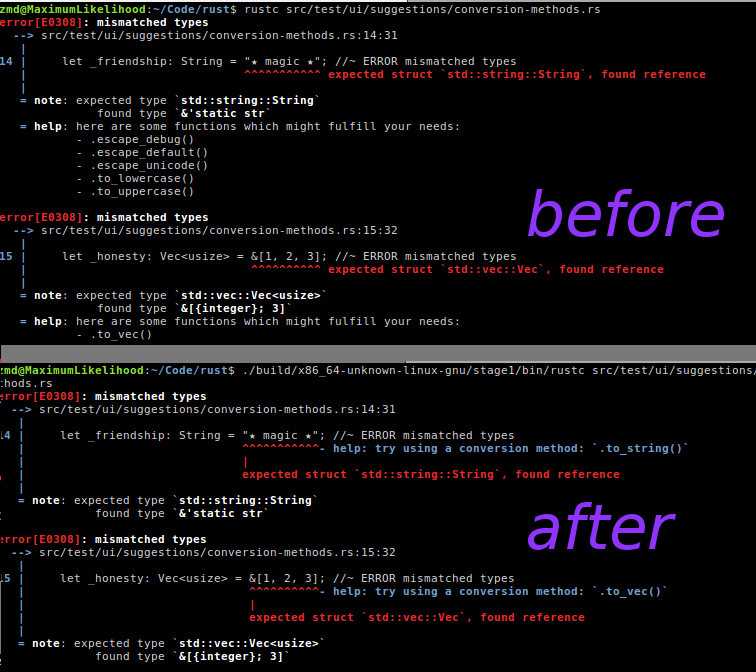
Previously, on a type mismatch (and if this wasn't preëmpted by a
higher-priority suggestion), we would look for argumentless methods
returning the expected type, and list them in a `help` note. This had two
major shortcomings: firstly, a lot of the suggestions didn't really make
sense (if you used a &str where a String was expected,
`.to_ascii_uppercase()` is probably not the solution you were hoping
for). Secondly, we weren't generating suggestions from the most useful
traits! We address the first problem with an internal
`#[rustc_conversion_suggestion]` attribute meant to mark methods that keep
the "same value" in the relevant sense, just converting the type. We
address the second problem by making `FnCtxt.probe_for_return_type` pass
the `ProbeScope::AllTraits` to `probe_op`: this would seem to be safe
because grep reveals no other callers of `probe_for_return_type`.
Also, structured suggestions are pretty and good for RLS and friends.
Unfortunately, the trait probing is still not all one would hope for: at a
minimum, we don't know how to rule out `into()` in cases where it wouldn't
actually work, and we don't know how to rule in `.to_owned()` where it
would. Issues #46459 and #46460 have been filed and are ref'd in a FIXME.
This is hoped to resolve#42929, #44672, and #45777.