This small fix should improve rust-analyzer resopnsivness for
real-time operations like onEnter handling.
Turns out, salsa's validation can take hundreds of milliseconds, and,
in case no changes were made, it won't be triggering any queries.
Because we check for cancellation in queries, that means that
validation is not cancellable!
What this PR does is injecting check_canceled checks into validation,
by using salsa's event API, which wasn't meant to be used like this,
but, hey, it works!
Here's the onEnter handling before and after this change:
https://youtu.be/7-ffPzgvH7o
1337: Move syntax errors our of syntax tree r=matklad a=matklad
I am not really sure if it's a good idea, but `SyntaxError` do not really belong to a `SyntaxTree`. So let's just store them on the side?
Co-authored-by: Aleksey Kladov <aleksey.kladov@gmail.com>
Very simple approach: For each identifier, set the hash of the range
where it's defined as its 'id' and use it in the VSCode extension to
generate unique colors.
Thus, the generated colors are per-file. They are also quite fragile,
and I'm not entirely sure why. Looks like we need to make sure the
same ranges aren't overwritten by a later request?
1299: Use ThemeColor and add support for light themes r=matklad a=lnicola
Part of #1294.
- switch to `ThemeColor`
- add light and high contrast theme definitions
- highlight control flow keywords and `unsafe`
Co-authored-by: Laurențiu Nicola <lnicola@dend.ro>
1208: [WIP] Goto for Macro's r=matklad a=Lapz
Adds goto definition for macros. Currently only works for macros in the current crate ~~otherwise it panics~~. Proper macro resolution needs to be added for it to resolve macros in other crates.
Todo
- [X] Allow goto from macro calls
- [X] Fix panics
- [x] Add tests
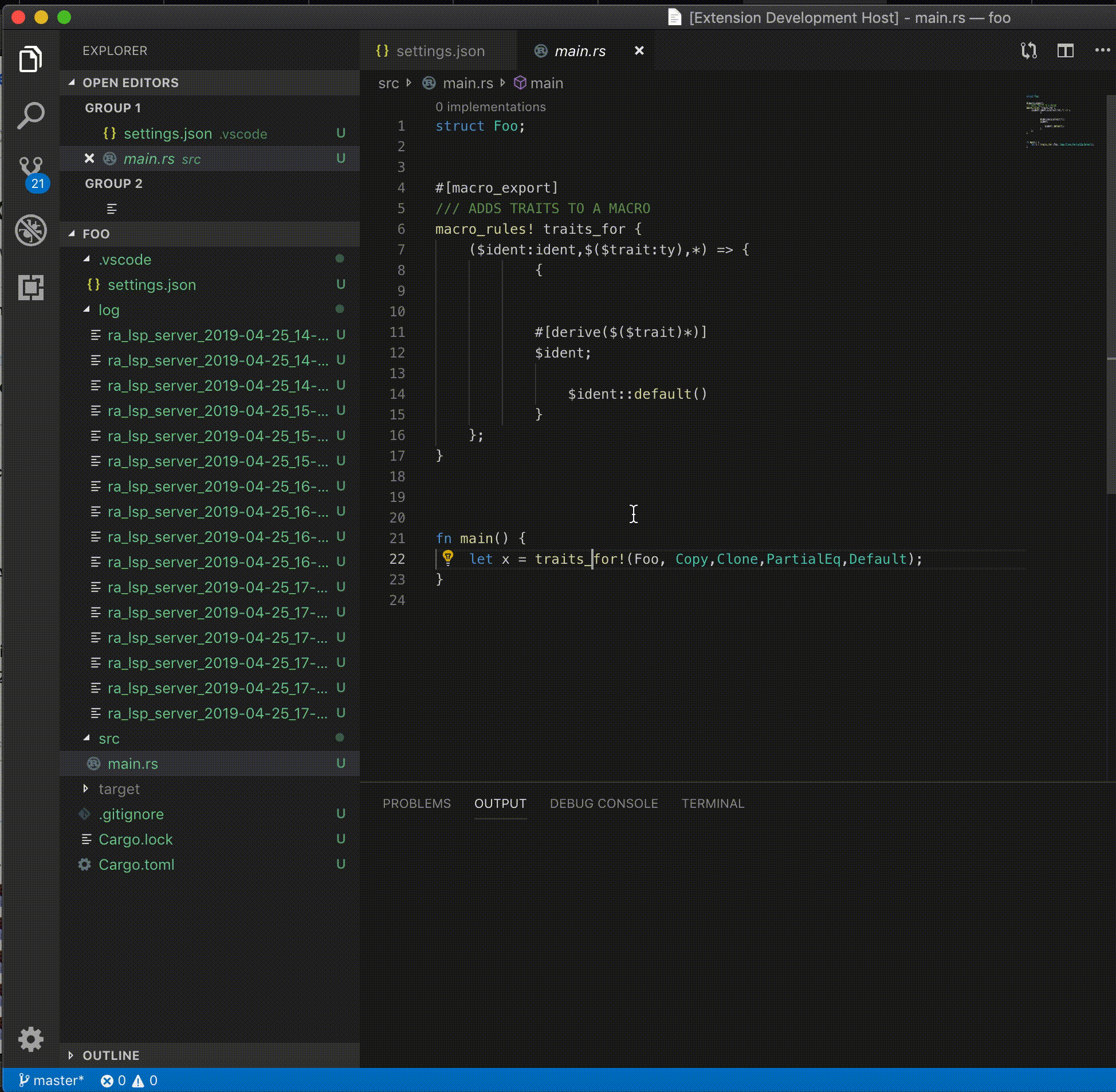
Co-authored-by: Lenard Pratt <l3np27@gmail.com>
E.g. in
```
let foo = 1u32;
if true {
<|>foo;
}
```
the hover shows `()`, the type of the whole if expression, instead of the more
sensible `u32`. The reason for this was that the search for an expression was
slightly left-biased: When on the edge between two tokens, it first looked at
all ancestors of the left token and then of the right token. Instead merge the
ancestors in ascending order, so that we get the smaller of the two possible
expressions.
1200: Allows searching for case-equivalent symbols (fixes#1151) r=matklad a=jrvidal
I couldn't find a nice, functional way of calculating the ranges in one pass so I resorted to a plain old `for` loop.
Co-authored-by: Roberto Vidal <vidal.roberto.j@gmail.com>
We really shouldn't be looking at the identifier at point. Instead,
all filtering and sorting should be implemented at the layer above.
This layer should probably be home for auto-import completions as
well, but, since that is not yet implemented, let's just stick this
into complete_scope.
This fixes the order in which candidates are chosen a bit (not completely
though, as the ignored test demonstrates), and makes autoderef work with trait
methods. As a side effect, this also makes completion of trait methods work :)
1067: Take number of arguments at the call-site into account for signature help r=matklad a=kjeremy
Fixes#1065
Co-authored-by: kjeremy <kjeremy@gmail.com>
1034: HIR diagnostics API r=matklad a=matklad
This PR introduces diagnostics API for HIR, so we can now start issuing errors and warnings! Here are requirements that this solution aims to fulfill:
* structured diagnostics: rather than immediately rendering error to string, we provide a well-typed blob of data with error-description. These data is used by IDE to provide fixes
* open set diagnostics: there's no single enum with all possible diagnostics, which hopefully should result in better modularity
The `Diagnostic` trait describes "a diagnostic", which can be downcast to a specific diagnostic kind. Diagnostics are expressed in terms of macro-expanded syntax tree: they store pointers to syntax nodes. Diagnostics are self-contained: you don't need any context, besides `db`, to fully understand the meaning of a diagnostic.
Because diagnostics are tied to the source, we can't store them in salsa. So subsystems like type-checking produce subsystem-local diagnostic (which is a closed `enum`), which is expressed in therms of subsystem IR. A separate step converts these proto-diagnostics into `Diagnostic`, by merging them with source-maps.
Note that this PR stresses type-system quite a bit: we now type-check every function in open files to compute errors!
Discussion on Zulip: https://rust-lang.zulipchat.com/#narrow/stream/185405-t-compiler.2Fwg-rls-2.2E0/topic/Diagnostics.20API
Co-authored-by: Aleksey Kladov <aleksey.kladov@gmail.com>
1031: Move most things out of ra_ide_api_light r=matklad a=detrumi
This moves everything except `structure` out of `ra_ide_api_light`. So this PR and #1019 finish up #1009, whichever is merged last should probably remove the `ra_ide_api_light` crate.
Also, `LocalEdit` was removed since it wasn't used any more.
Co-authored-by: Wilco Kusee <wilcokusee@gmail.com>
1021: Wasm dependencies r=matklad a=detrumi
As a first step towards running RA on WASM (see #1007), this tweaks the dependencies somewhat so that projects built using `wasm-pack` can use `ra_ide_api` as a dependency.
There were two problems:
- use of undeclared type or module `MmapInner`
This error occurred because of the `memmap` crate, as a dependency of `fst`
Solution: specify `default-features = false` for the `fst` package (see https://github.com/BurntSushi/fst/issues/70)
- use of undeclared type or module `imp`
This happened in the `wait-timeout` crate ([which uses `Command` under the hood](https://github.com/alexcrichton/wait-timeout/issues/18)), a dependency of `rusty-fork` which is a dependency of `proptest`.
Solution: move `proptest` to dev-dependencies and add `#[cfg(test)]` to the `test_utils` crate.
**Edit:** Oh, that causes trouble with resolving the import when running the tests. Hmm...
Co-authored-by: Wilco Kusee <wilcokusee@gmail.com>
We simply remove all the CUSTOM_MARKERS before attempting to parse the file.
This allows for the syntax selection to work with most of the test strings.
This allows us to select a string or portions of it and try parsing it as rust
syntax. This is mostly helpful when developing tests where the test
itself contains some rust syntax as a string.
908: Enable markup for hover on expressions which resolve using type_of r=matklad a=vipentti
This adds highlighting when hovering over items which are resolved using
`type_of`.
This adds basic highlighting, discussed in #904.
Co-authored-by: Ville Penttinen <villem.penttinen@gmail.com>
This contains the syntax range of the name itself, allowing NavigationTarget to
properly set the focus_range. This should make it so that when using symbol
based navigation, we should always focus on the name, instead of the full range.
866: Implement basic support for Associated Methods r=flodiebold a=vipentti
This is my attempt at learning to understand how the type inference works by adding basic support for associated methods. Currently it does not resolve associated types or constants.
The basic idea is that `Resolver::resolve_path` returns a new `PathResult` type, which has two variants, `FullyResolved` and `PartiallyResolved`, fully resolved matches the previous behavior, where as `PartiallyResolved` contains the `PerNs<Resolution` in addition to a `segment_index` which contains the index of the segment which we failed to resolve. This index can then be used to continue inference in `infer_path_expr` using the `Type` we managed to resolve.
This changes some of the previous apis, so looking for feedback and suggestions.
This should enable fixing #832
Co-authored-by: Ville Penttinen <villem.penttinen@gmail.com>
This also adds new pub(crate) resolve_path_segments which returns the
PathResult, which may or may not be fully resolved. PathResult is also now
pub(crate) since it is an implementation detail.
864: Fix handling of generics in tuple variants and refactor a bit r=matklad a=flodiebold
(The problem was that we created separate substitutions for the return value, so we lost the connection between the type arguments in the constructor call and the type arguments of the result.)
Also make them display a tiny bit nicer.
Fixes#860.
Co-authored-by: Florian Diebold <flodiebold@gmail.com>
Co-authored-by: Florian Diebold <florian.diebold@freiheit.com>
This is done in `infer_path_expr`. When `Resolver::resolve_path` returns
`PartiallyResolved`, we use the returned `Resolution` together with the given
`segment_index` to check if we can find something matching the segment at
segment_index in the impls for that particular type.
844: Refactor find_all_refs to return ReferenceSearchResult r=vipentti a=vipentti
This refactors `find_all_refs` to return a new `ReferenceSearchResult` based on feedback in #839.
There are few questions/notes regarding the refactor:
1. Introducing `NavigationTarget::from_bind_pat` this simply forwards the call to `NavigationTarget::from_named`, could we just expose `from_named` directly as `pub(crate)` ?
2. Added an utility method `NavigationTarget::range` since there were few places where you would use `self.focus_range.unwrap_or(self.full_range)`
3. Implementing `IntoIterator` for `ReferenceSearchResult`. This turns `ReferenceSearchResult` into an iterator over `FileRanges` and allows previous code to mostly stay as it was based on the order that `find_all_refs` previously had (declaration first and then the references). I'm not sure if there is a way of doing the conversion to `IntoIter` without the allocation of a new vector
4. Is it possible to have a binding without a name? I'm not sure if the `NavigationTarget::from_bind_pat` can cause some edge-cases that previously were ok
This fixes#835.
Co-authored-by: Ville Penttinen <villem.penttinen@gmail.com>
This makes it more like the other code model types.
Also make Module::definition_source/declaration_source return HirFileIds, to
make them more like the other source functions.