They can be derived directly from the `hir::Item`, there's no special
logic.
- TypeDef
- OpaqueTy
- Constant
- Static
- TraitAlias
- Enum
- Union
- Struct
lint: Do not provide suggestions for non standard characters
Fixes#77273
Only provide suggestions if the case-fixed result is different than the original.
rustc_expand: Mark inner `#![test]` attributes as soft-unstable
Custom inner attributes are feature gated (https://github.com/rust-lang/rust/issues/54726) except for attributes having name `test` literally, which are not gated for historical reasons.
`#![test]` is an inner proc macro attribute, so it has all the issues described in https://github.com/rust-lang/rust/issues/54726 too.
This PR gates it with the `soft_unstable` lint.
Reworks Sccc computation to iteration instead of recursion
Linear graphs, producing as many scc's as nodes, would recurse once for every node when entered from the start of the list. This adds a test that exhausted the stack at least on my machine with error:
```
thread 'graph::scc::tests::test_deep_linear' has overflowed its stack
fatal runtime error: stack overflow
```
This may or may not be connected to #78567. I was only reminded that I started this rework some time ago. It might be plausible as borrow checking a long function with many borrow regions around each other—((((((…))))))— may produce the linear list setup to trigger this stack overflow ? I don't know enough about borrow check to say for sure.
This is best read in two separate commits. The first addresses only `find_state` internally. This is classical union phase from union-find. There's also a common solution of using the parent pointers in the (virtual) linked list to track the backreferences while traversing upwards and then following them backwards in a second path compression phase.
The second is more involved as it rewrites the mutually recursive `walk_node` and `walk_unvisited_node`. Firstly, the caller is required to handle the unvisited case of `walk_node` so a new `start_walk_from` method is added to handle that by walking the unvisited node if necessary. Then `walk_unvisited_node`, where we would previously recurse into in the missing case, is rewritten to construct a manual stack of its frames. The state fields consist of the previous stack slots.
Arena: use specialization to avoid copying data
In several cases, a `Vec` or `SmallVec` is passed to `Arena::alloc_from_iter` directly. This PR makes sure those cases don't copy their data unnecessarily, by specializing the `alloc_from_iter` implementation.
This commit updates the `library/backtrace` submodule which primarily
pulls in support for split-debuginfo on macOS, avoiding the need for
`dsymutil` to get run to get line numbers and filenames in backtraces.
Downgrade the await holding lints from correctness
We found a false positive in these lints (see https://github.com/rust-lang/rust-clippy/issues/6353 for more details). As a short-term mitigation, this downgrades the lints from correctness to limit the noise.
changelog: downgrade AWAIT_HOLDING_REFCELL_REF and AWAIT_HOLDING_LOCK to pedantic. From rustup earlier, where I forgot the changlog: deprecate [`panic_params`] (uplifted)
BTreeMap: replace Root with NodeRef<Owned, ...>
`NodeRef<marker::Owned, …>` already exists as a representation of root nodes, and it makes more sense to alias `Root` to that than to reuse the space-efficient `BoxedNode` that is oblivious to height, where height is required.
r? `@Mark-Simulacrum`
unix/weak: pass arguments to syscall at the given type
Given that we know the type the argument should have, it seems a bit strange not to use that information.
r? `@m-ou-se` `@cuviper`
Never inline naked functions
The `#[naked]` attribute disabled prologue / epilogue emission for the
function and it is responsibility of a developer to provide them. The
compiler is no position to inline such functions correctly.
Disable inlining of naked functions at LLVM and MIR level.
Closes#60919.
Add lint for panic!("{}")
This adds a lint that warns about `panic!("{}")`.
`panic!(msg)` invocations with a single argument use their argument as panic payload literally, without using it as a format string. The same holds for `assert!(expr, msg)`.
This lints checks if `msg` is a string literal (after expansion), and warns in case it contained braces. It suggests to insert `"{}", ` to use the message literally, or to add arguments to use it as a format string.
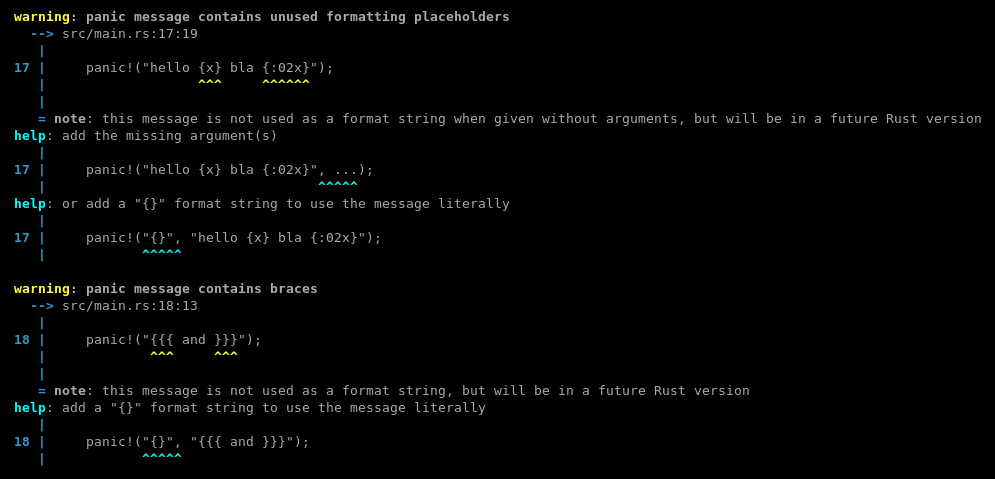
This lint is also a good starting point for adding warnings about `panic!(not_a_string)` later, once [`panic_any()`](https://github.com/rust-lang/rust/pull/74622) becomes a stable alternative.
Rollup of 11 pull requests
Successful merges:
- #79119 (Clarify availability of atomic operations)
- #79123 (Add u128 and i128 integer tests)
- #79177 (Test drop order for (destructuring) assignments)
- #79181 (rustdoc: add [src] links to methods on a trait's page)
- #79183 (Make compiletest testing use the local sysroot)
- #79185 (expand/resolve: Pre-requisites to "Turn `#[derive]` into a regular macro attribute")
- #79193 (Revert #78969 "Normalize function type during validation")
- #79194 (Make as{_mut,}_slice on array::IntoIter public)
- #79204 (Add jyn514 email alias to mailmap)
- #79212 (Move `rustc_ty` -> `rustc_ty_utils`)
- #79217 (Add the "memcpy" doc alias to slice::copy_from_slice)
Failed merges:
r? `@ghost`
`@rustbot` modify labels: rollup
The `#[naked]` attribute disabled prologue / epilogue emission for the
function and it is responsibility of a developer to provide them. The
compiler is no position to inline such functions correctly.
Disable inlining of naked functions at LLVM and MIR level.
Make as{_mut,}_slice on array::IntoIter public
The functions are useful in cases where you want to move data out of the IntoIter in bulk, by transmute_copy'ing the slice and then forgetting the IntoIter.
In the compiler, this is useful for providing a sped up IntoIter implementation. One can alternatively provide a separate allocate_array function but one can avoid duplicating some logic by passing everything through the generic iterator using interface.
As per suggestion in https://github.com/rust-lang/rust/pull/78569/files#r526506964